fetch()
What is fetch()
It’s part of the JavaScript Web API
The fetch() function is a modern JavaScript API used to make network requests, primarily over HTTP and HTTPS. It allows developers to retrieve or send resources such as JSON data, text, or files from web servers. Unlike its predecessor, XMLHttpRequest, fetch() is Promise-based, making it easier to work with asynchronous requests and handle responses more cleanly. It’s widely used for interacting with RESTful APIs, loading resources dynamically, and fetching data without reloading the web page.
When you call fetch(), it returns a Promise that resolves to the Response object. This object contains information about the request and response, such as headers, status codes, and the response body. Developers can then chain methods like .json(), .text(), or .blob() on the Response object to parse the content into the desired format. For example, JSON data fetched from an API can be easily converted into a JavaScript object using .json(). In addition to GET requests, fetch() also supports POST, PUT, DELETE, and other HTTP methods, allowing for full control over API interactions.
One of the major benefits of fetch() is its cleaner and more readable syntax compared to older methods like XMLHttpRequest. With the addition of async/await syntax in JavaScript, fetch() has become even more powerful, enabling developers to write code that looks synchronous but still handles asynchronous requests effectively. This allows for better error handling, more intuitive control flow, and a more modern approach to working with web data. However, developers still need to handle potential errors carefully, as fetch() doesn’t automatically reject for HTTP errors like 404 or 500.
Not that the fetch() API, the function name and parameter list is consistent (the same across all environment, vanilla js, nodejs and so on), but how the response object is packaged are usually not the same. The fields may differ! In other words, the node.js response object may differ from the build in browser.
Differences in Response Object Handling
- In the browser, the response object is straightforward:
- Access the response status with
response
.status
- Access headers with
response
.headers.get('content-type')
- Parse the body using
response.json()
,response.text()
, orresponse.blob()
.All these function return a promise object
- Access the response status with
- In Node.js (depending on the version and library):
- Node.js 18+: The response will be similar to the browser’s native implementation since it now includes a built-in
fetch
. - Node.js <18 with
node-fetch
orundici
: The response may involve streams (e.g.,response.body
might be aReadableStream
) and additional methods like.buffer()
to handle the body data.
- Node.js 18+: The response will be similar to the browser’s native implementation since it now includes a built-in
Summary
- Request call: The method signature and options are the same in both environments, ensuring consistency.
- Response object: While the core properties of the response object are the same, the underlying packaging (e.g., headers stored as symbols, body handling via streams) may differ depending on the environment—browser vs. Node.js.
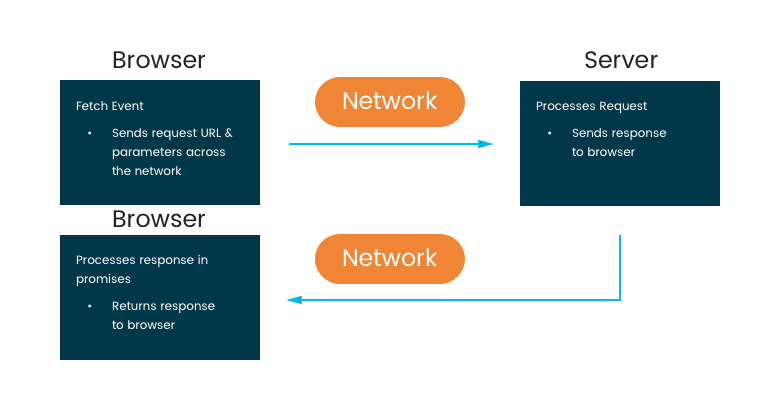
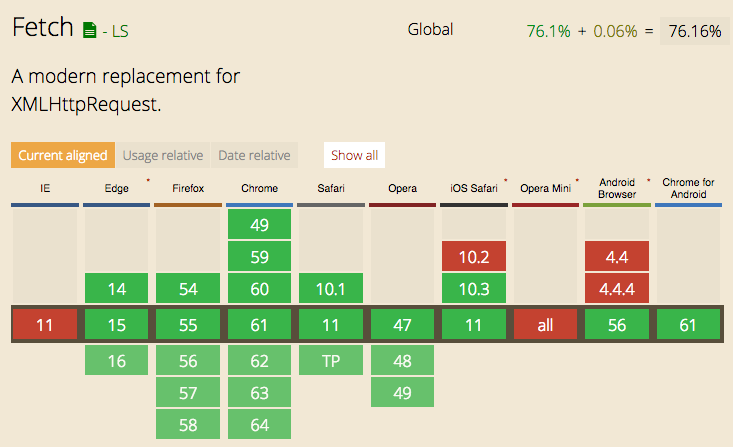
How to install a fetch
NO!
The Web APIs, including fetch() they are built into the browser environment. As you are running your JavaScript code in a modern web browser, you can directly use these APIs without any additional setup or installation. The browser provides the necessary functionality. This is one of the key advantages of using Web APIs.
Termomonlogy and Concept
-
- Promise:
- An object representing the eventual completion or failure of an asynchronous operation. fetch() returns a Promise that resolves to a Response object.
- Response Object:
- The object returned by the fetch() function that contains information about the request and its response, including status, headers, and methods to read the response body..
- Request Method:
- The HTTP method used for the request, such as GET, POST, PUT, DELETE, etc. This can be specified in the options of the fetch() call
- Headers:
- Key-value pairs sent with HTTP requests and responses that provide additional context or metadata, such as content type or authentication tokens.
- CORS (Cross-Origin Resource Sharing):
- A security feature implemented in browsers that restricts web pages from making requests to a different domain than the one that served the web page, unless the server explicitly allows it.
- JSON:
- JavaScript Object Notation, a lightweight data interchange format that is commonly used with fetch() for APIs. The .json() method of the Response object parses the response body as JSON
- Error Handling:
- The process of managing errors that may occur during the fetch operation, including network errors and HTTP errors, which requires checking the ok property of the Response object.
- Async/Await:
- A syntactic feature in JavaScript that allows you to write asynchronous code in a more synchronous fashion, making it easier to read and manage Promises returned by fetch().
- AbortController:
- An interface that allows you to abort a fetch request if needed, giving you more control over long-running requests.
- Body (aka payload):
- The content (the actual useful data – NOT all metadata) sent with the request/response, particularly for POST and PUT requests. You can send data in various formats, like JSON, FormData, or plain text.
- Promise:
HTTP Headers
The header are data that are sent together with the payload everytime a request is made. There are some standard headers and some non-standard BUT commonly adopted “extensions”
Standard Headers
- Content-Type
- Authorization
- Accept
- Cache-Control
- User-Agent
- Accept-Encoding
Non-standard
- X-Requested-With
- Cookie
The basics
the fetch() function
Note 1:works in hand in hand with the object/class promise
-
- Always return a
promise
object- Could be in 1 of 3 states
pending
- This is the initial state, mean that the operation(asynchrounous) is still ongoing.Will transition to fulfilled or rejected
fulfilled
- The operation is successful. At this state the promise object has a resulting return value which can be accessed in the
.then()
method
- The operation is successful. At this state the promise object has a resulting return value which can be accessed in the
rejected
- The operation is failed. The promise object has a reason for the failure and than can be accessed in
.catch()
method
- The operation is failed. The promise object has a reason for the failure and than can be accessed in
- Once a promise is either fulfilled or rejected, it can NOT change state again
- Supports chaining
.catch()
and.then()
- Could be in 1 of 3 states
- Asyncchrounus execution
- The code continues to execute line by line if the while the fetch() operation occours
- Always return a
Code that uses Promise
EXAMPLE 1
const fetchData = new Promise((resolve, reject) => {
console.log('Inside the Promise executor function'); // This runs immediately
const data = { id: 1, name: 'Product A' };
resolve(data); // This resolves the promise immediately
});
fetchData.then((result) => {
console.log('Data received:', result); // This runs asynchronously when the promise is fulfilled
});
Code that uses Promise
EXAMPLE 2
const fetchData = new Promise((resolve, reject) => {
console.log('Starting async operation...'); // Runs immediately
// Simulating an asynchronous operation (e.g., fetching data from an API)
setTimeout(() => {
const data = { id: 1, name: 'Product A' };
resolve(data); // Resolves after 2 seconds
}, 2000);
});
fetchData.then((result) => {
console.log('Data received:', result); // Runs after promise is resolved
});
node.js
fetch()
In it’s basic form it takes two parameters. The first parameter is mandatory and the seconds is optional. And it ALWAYS returns a promise.
Note:You could use a single argument and passing a Request object
//General form
fetch(parameterOneIsFullUrl, optionsToRequest);
Example 1
In it’s basic form it takes two parameters. The first parameter is mandatory and the seconds is optional. And it ALWAYS returns a promise.
const fullUrl = 'https://restcountries.com/v3.1/all';
//Default to a GET(missing second parameter),returnsa promise object
const responseObject = fetch(fullUrl);
//Will probably display the status pending for the promise object
console.log(responseObject);
The previous code is impractical because you need to use it together with .then()
and .catch()
member functions of the promise object
Example 2
The whole response object
function printRawResponseObject(){
//Print the response objext
responsePromise
.then(responseObject => {
console.log(responseObject);
})
.catch(error =>{
console.log("Something went wrong:");
console.log(error);
});
}
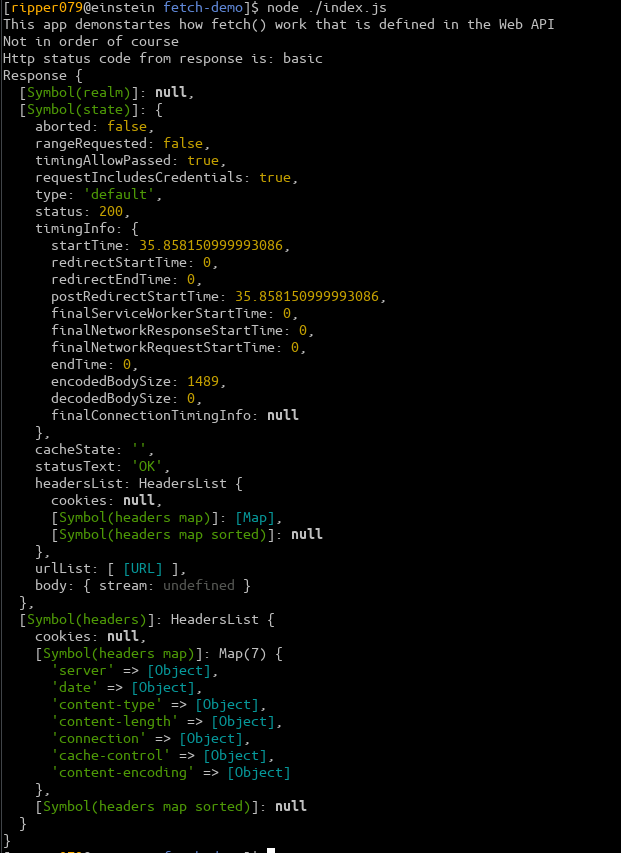
Example 3
The whole response object headers
function printResponseHeadersObject(){
//Get the header of the response
fetch(fullUrl)
.then(responseObject => {
//Actually return instance of an interface
const headersObject = responseObject.headers;
console.log(headersObject);
})
.catch(errorResponse => {
});
}
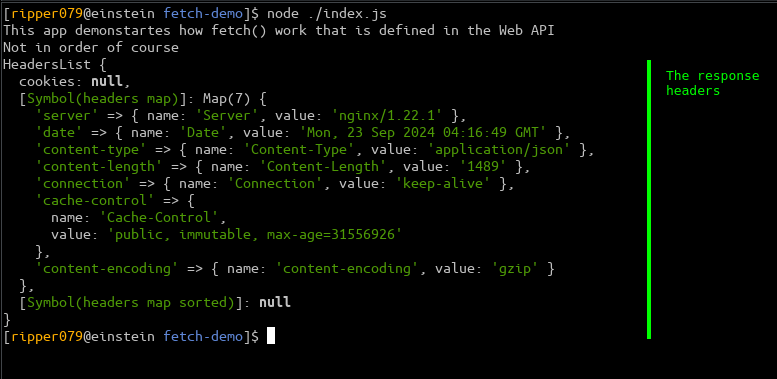
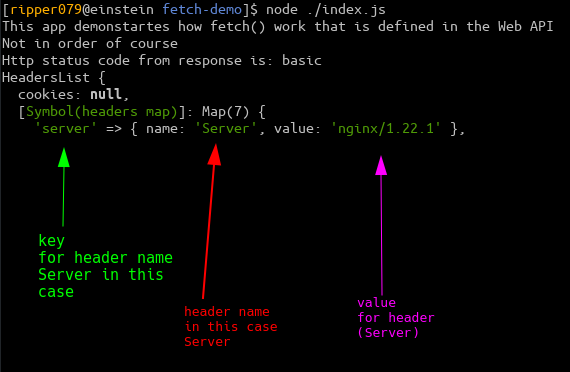
Example 4
This print all the headers of the response
function printAllHeadersFromResponse()
{
fetch(fullUrl)
.then(responseObj => {
//Iterate over all objects
const headersObject = responseObj.headers;
headersObject.forEach((value, key) => {
console.log("Key is: " + key + " and value is: " + value);
});
})
.catch(responseError => {
console.log("There was an error");
console.log(responseError);
});
}
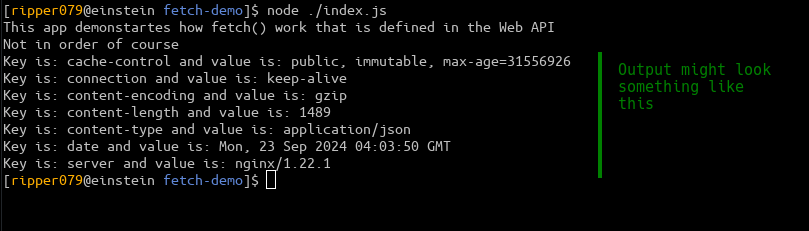
Note: The content-length unit is byte
Example 5
This print value of the header server
function printHeaderServer(){
fetch(fullUrl)
.then(responseObj => {
//Common headers are
//server, date, content-type, content-length, content-encoding...
const headersObject = responseObj.headers;
if (headersObject.has('server')){
console.log("server header is present");
console.log(headersObject.get('server'));
}
else {
console.log("server header NOT present");
}
}
)
.catch (responseErr => {
console.log("Something went wrong");
console.log(responseErr);
});
}
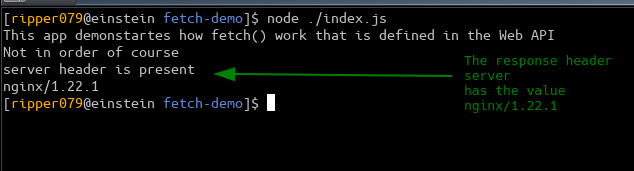
Example 6
This print value of the response http code
function printStatusCodeForEachGroup(){
fetch(fullUrl)
.then(responseObj => {
//Status code for the response is in the responseObj
console.log("Http status code from response is: " + responseObj.status);
// Informational responses (status 100–199)
if (responseObj.status >= 100 && responseObj.status < 200)
{
console.log('Informational response:', responseObj.status);
}
// Successful responses (status 100–199)
else if (responseObj.status >= 200 && responseObj.status < 300)
{
console.log('Successful response:', responseObj.status);
}
// Redirection responses (status 300–399)
else if (responseObj.status >= 300 && responseObj.status < 400)
{
console.log('Redirection response:', responseObj.status);
}
// Client errors (status 400–499)
else if (responseObj.status >= 400 && responseObj.status < 500)
{
console.log('Client error:', responseObj.status);
}
// Server errors (status 500–599)
else if (responseObj.status >= 500 && responseObj.status < 600)
{
console.log('Server error:', responseObj.status);
}
})
.catch(resonseErrObj => {
console.log("Something went wrong...");
console.log(resonseErrObj);
});
}
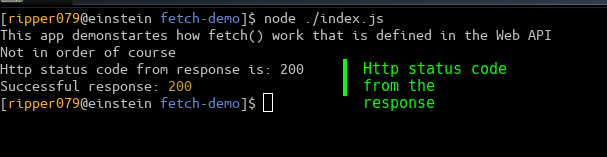
Example 7
This uses a Request object when fetching
const request = new Request('https://api.example.com/data', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer your-token-here'
},
body: JSON.stringify({ key: 'value' }) // For POST, PUT, PATCH methods
});
fetch(request)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Flowchart - Promise
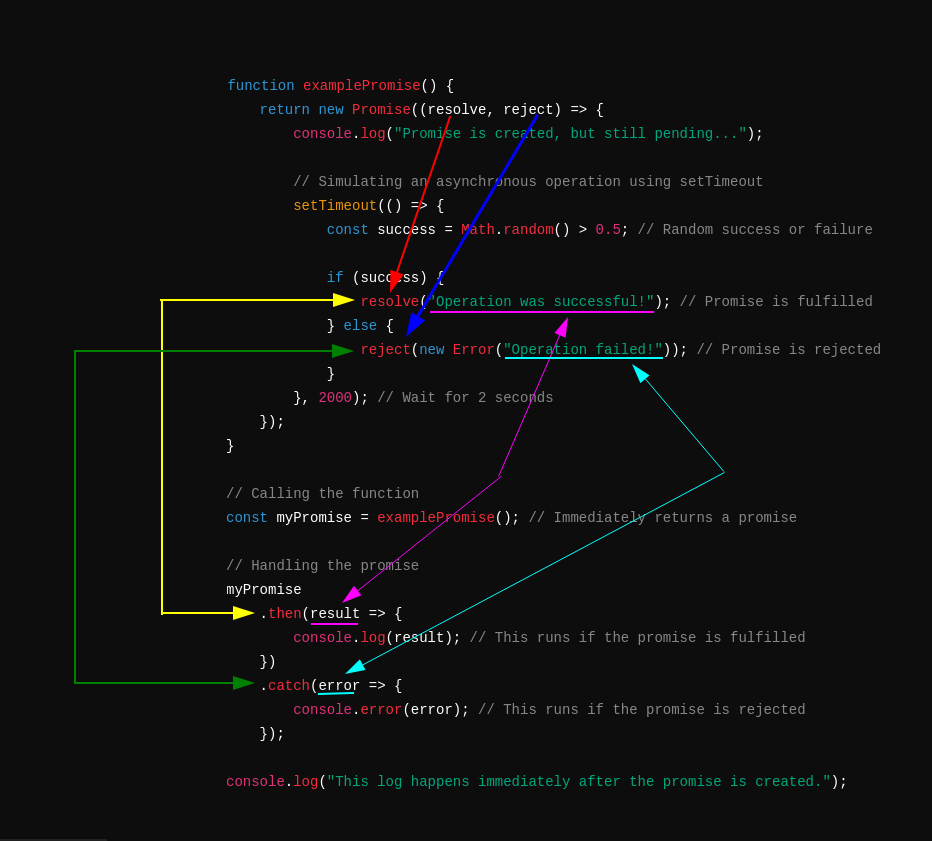