Git
What is Git
Git is a distributed version control system that enables you to manage code changes efficiently in collaborative software development projects using the git command.
A committed change, also known as a git commit, can be applied in various scenarios, including implementing new features, fixing bugs, and more.
Git commits are essential for tracking code modifications, ensuring collaboration among team members, and maintaining a well-organized codebase.
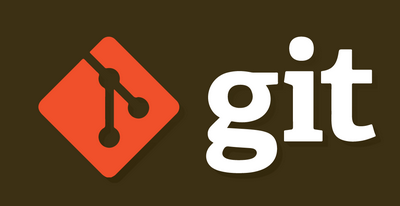
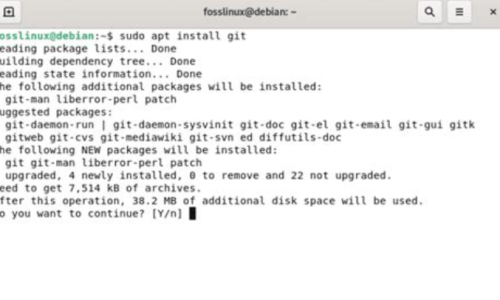
How to install git
Install the package
sudo apt install git
Verify git is is installed
git -v
Termomonlogy and Concept
-
Default Branch:
Git:
- Still “master”
- Options for other default branch names
Github:
- Default now “main” (new repos only)
- Can be reset back to “master”
Ignore files:
Git:
- Is made in the file .gitignore
Github View - HTTPS
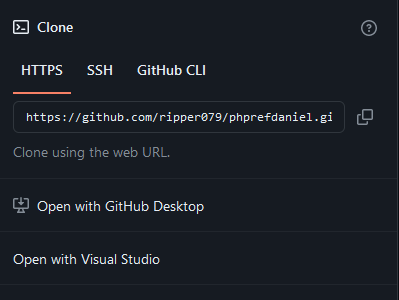
# git remote set-url origin
where origin is
git@github.com:[Gitowner]/[reponame].git
E.i (with user and password)
https://github.com/ripper079/phprefdaniel.git
E.i (with public/private keys)
git@github.com:ripper079/phprefdaniel.git
With password
git remote set-url https://github.com/ripper079/phprefdaniel.git
With keys
git remote set-url git@github.com:ripper079/phprefdaniel.git
Github View - SSH
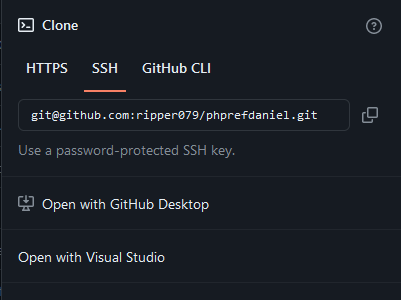
Commands - INIT
DESCRIPTION | COMMAND |
---|---|
Initialize git Set up necessary data structures and files, creating a hidden subfolder (.git) |
git init |
Initializes a new Git repository specifically in a directory called "demo-one." This means that Git will set up its version control system in the "demo-one" folder |
git init demo-one |
initializes a new Git repository with the name "demo-one" and sets the default branch to "main." |
git init -b main demo-one |
Clone a repo into the current folder User/Pass |
git clone https://github.com/ripper079/phprefdaniel.git |
Clone a repo into the current folder Public/Private ket |
git clone git@github.com/ripper079/phprefdaniel.git |
Commands - Staging and Commit
DESCRIPTION | COMMAND |
---|---|
Add all changes in the working directory to the staging area. | git add . |
Stage all changes, including new, modified, and deleted files | git add -A |
Displays information about the current state of your Git repository Display the current state of the working directory and the staging area |
git status |
Commit all changes with a commit message. | git commit -a -m "Commit message" |
Reset to a Previous Commit Soft Reset: Moves the branch pointer and keeps changes in the staging area |
git reset --soft "commit-hash" |
Reset to a Previous Commit Mixed Reset: Moves the branch pointer and keeps changes in the working directory (unstaged). |
git reset --mixed "commit-hash" |
Reset to a Previous Commit Hard Reset: Moves the branch pointer and discards all changes in the working directory and staging area. |
git reset --hard "commit-hash" |
Commands - Remote
DESCRIPTION | COMMAND |
---|---|
List all remote repositories associated with the current repository. | git remote |
Display more detailed information about the remote repositories, including their URLs. | git remote -v |
Add a new remote repository with the name "origin" and the specified URL | git remote add origin "remote_url" |
Remove the remote repository named "origin" | git remote rm origin |
Change Remote Push URL ( git remote set-url --push origin https://github.com/ripper079/referenceJS.git ) ( git remote set-url --push origin git@github.com:ripper079/referenceJS.git ) |
git remote set-url --push "remotename" "new_fetch_pull_url" |
Change Remote Fetch/Pull URL (git remote set-url origin https://github.com/ripper079/referenceJS.git ) ( git remote set-url origin git@github.com:ripper079/referenceJS.git ) |
git remote set-url "remotename" "new_fetch_pull_url" |
Rename a remote repository from old_name to new_name | git remote rename old_name new_name |
Display information about a specific remote repository named "origin" | git remote show origin |
Fetch the latest changes from all remote repositories | git remote update |
Change the URL of the remote repository named "origin." | git remote set-url origin |
Display help information about the git remote command. | git remote -h |
Get the URL of the remote repository named "origin." | git config --get remote.origin.url |
Fetches changes from a remote repository and merges them into the current branch. Pulls data from the specified remote (often origin by default) |
git pull |
Retrieves changes from a remote repository without merging them into your current branch. Fetches data from the specified remote (often origin by default). |
git fetch |
Sends your local commits to a remote repository Pushes data to the specified remote (often origin by default). |
git push |
If you have a local branch named feature and you want to push it to the feature branch on the collaborator remote, you would run |
git push -u collaborator feature |
Commands - Branches
DESCRIPTION | COMMAND |
---|---|
List all local branches in the repository | git branch |
Create a new branch named branch_name. | git branch branch_name |
Delete a specific branch (branch_name) after its changes have been merged. | git branch -d branch_name |
Rename the current branch to new_branch_name. | git branch -m new_branch_name |
List all local and remote branches. | git branch -a |
List all remote branches | git branch -r |
Create a new branch (new_branch) based on the current branch | git branch -c new_branch |
CONFIG This Git command sets the default branch name for newly initialized repositories on your system. In this case, it configures Git to use "main" as the default branch name. |
git config --global init.defaultBranch main |
Move/Swith to branch branchname |
git checkout "branchname" |
Move/Swith to previous commit Note :Puts you in detached HEAD state. Return to last commit in a branch with git checkout "branchname" |
git checkout "commit-hash" |
Move/Swith to previous commit Note :Puts you in detached HEAD state. Return to last commit in a branch with git checkout "branchname" |
git checkout "commit-hash" |
Default branch are usually called main
OR master
Commands - MiSC
DESCRIPTION | COMMAND |
---|---|
Display the current configuration settings for the repository. | git config --list |
Display the current global configuration settings for git | git config --global --list |
Set the default text editor for Git commit messages. | git config core.editor "editor" |
Enable colored output in the Git command-line interface. | git config color.ui auto |
Get the URL of the remote repository named "origin." | git config --get remote.origin.url |
Display current git version | git version |
Shows a chronological list of commits made to the repository | git log |
View the commit history in a single line format | git log --oneline |
Display a graphical representation of the commit history | git log --graph --oneline |
User Commands
DESCRIPTION | COMMAND |
---|---|
Set the user name for your commits in local repository.
|
git config user.name "Your Name" |
Set the email address for your commit in local repository |
git config user.email "your.email@example.com" |
Set the global user name for all Git repositories on your system. |
git config --global user.name "Your Name" |
Set the global email address for all Git repositories on your system. |
git config --global user.email "your.email@example.com" |
Remove the user name configuration for the repository. | git config --unset user.name |
Remove the email configuration for the repository. | git config --unset user.email |
Remove the global user name configuration. | git config --unset user.name |
Remove the global user email configuration. | git config --global --unset user.email |
Display the current configuration settings for the repository. | git config --list |
Displays the global configured username for Git Applies to for all repositories on the system for the current user. |
git config --global user.name |
Displays the global configured email for Git Applies to for all repositories on the system for the current user. |
git config --global user.email |
Displays the specific repository configured username for Git Applies to for local repositories on the system for the current user. |
git config user.name |
Displays the specific repository configured email for Git Applies to for local repositories on the system for the current user. |
git config user.email |
When setting a local username/email it overrides the global setting for username/email
.gitignore
# Logs
logs
*.log
npm-debug.log*
yarn-debug.log*
yarn-error.log*
lerna-debug.log*
# Diagnostic reports (https://nodejs.org/api/report.html)
report.[0-9]*.[0-9]*.[0-9]*.[0-9]*.json
# Runtime data
pids
*.pid
*.seed
*.pid.lock
# Directory for instrumented libs generated by jscoverage/JSCover
lib-cov
# Coverage directory used by tools like istanbul
coverage
*.lcov
# nyc test coverage
.nyc_output
# Grunt intermediate storage (https://gruntjs.com/creating-plugins#storing-task-files)
.grunt
# Bower dependency directory (https://bower.io/)
bower_components
# node-waf configuration
.lock-wscript
# Compiled binary addons (https://nodejs.org/api/addons.html)
build/Release
# Dependency directories
node_modules/
jspm_packages/
# TypeScript v1 declaration files
typings/
# TypeScript cache
*.tsbuildinfo
# Optional npm cache directory
.npm
# Optional eslint cache
.eslintcache
# Microbundle cache
.rpt2_cache/
.rts2_cache_cjs/
.rts2_cache_es/
.rts2_cache_umd/
# Optional REPL history
.node_repl_history
# Output of ‘npm pack’
*.tgz
# Yarn Integrity file
.yarn-integrity
# dotenv environment variables file
.env
.env.test
# parcel-bundler cache (https://parceljs.org/)
.cache
# Next.js build output
.next
# Nuxt.js build / generate output
.nuxt
dist
# Gatsby files
.cache/
# Comment in the public line in if your project uses Gatsby and *not* Next.js
# https://nextjs.org/blog/next-9-1#public-directory-support
# public
# vuepress build output
.vuepress/dist
# Serverless directories
.serverless/
# FuseBox cache
.fusebox/
# DynamoDB Local files
.dynamodb/
# TernJS port file
.tern-port
src/settings.json
.DS_Store
Workflow of git
Create from scratch
1.Start by creating a repository remotely on e.i github, gitea…
a) Make sure to note/write the remote repository, https and ssh usually
https://github.com/ripper079/bookbest.git
or
git@github.com:ripper079/bookbest.git
Note 1: https://github.com/[Gitowner]/[reponame].git
Note 2: git@github.com:[Gitowner]/[reponame].git
2. Create a repository locally on the computer
git init
3. Set up git user details (either globally or locally) [This step might be optional]
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
4. Create some file(s) and edit them. Save them
echo "# bokenbast" >> README.md
5. Stage changes
git add .
6. Commit changes
git commit -m "Initial commit message"
7. Rename the current branch to the default branch on the remote repository. Usually it’s master or main. Lets play that the default branch is main
git branch -M main
8. Add a newRemote Repository – (HTTP)
a) git remote add origin https://github.com/yourusername/your-repo.git
OR (SSH)
b) git remote add origin git@github.com:yourusername/your-repo.git
c) Verify that remote is setup correctly with git remote -v
9. Push to remote repository
git push -u origin main
Note 1: This is done once, setting up tracking relationship. Subsequent push call be made with git push
Note 2: Its actually possible to set up multiple remotes
Note 3: The -u option is setting up the tracking relationship
Create a new branch
1. Navigate to the git respositry (local computer), where the .git folder is located
2 a) Run the command
git checkout -b "branch-name"
This code creates the branch and also switched to it
OR
b) Run the commands
git branch "branch-name"
git checkout "branch-name"
First command creates the branch and the seconds one switches to it
Continue work
1. Switch to the branch
git checkout main
Switches to the branch main
2. Pull latest changes form remote remote repository
git pull origin main
3. Make your changes: Edit files, add new features or fix bugs as needed
4. Stage your changes. Add modified or new files to the staging area
git add .
5. Commit changes
git commit -m "Describe your changes"
6. Pull again (optional but recommended). It’s a good practice to pull again before pushing, especially if other team members are pushing changes frequently. This helps avoid conflicts.
git pull origin main
7. Resolve and merge conflicts (if applicable)
git add "conflicted-file"
git commit -m "Resolve conflicts"
8. Push your changes to the remote repository
git push origin main
Git conflicts
1. Fetch the Latest Changes: Start by fetching the latest changes from the remote repository to ensure you have the most up-to-date commits
git fetch origin
2. Check the Differences: It’s a good idea to see what changes are in the remote main branch compared to your local branch. You can use
git diff HEAD origin/main
3 a) Merge (or Rebase) the Remote Changes
Merge
git merge origin/main
b) If there are merge conflicts, resolve them manually in the affected files. Open then in a text editor
c) After resolving conflicts, run
git add .
git commit -m "A proper message enter here"
4. Push Your Changes Again
git push origin main