WP_Query
What is WP_Query
In the context of WordPress, “WP_Query” is a class used to query posts from the WordPress database. It’s a powerful tool that allows developers to retrieve posts or custom post types based on various parameters such as post type, category, tag, author, date, and more.
The WP_Query class is defined in the file /wp-includes/class-wp-query.php
One of the best way to play with WP_Query is to make a page that is using a layout template! Create a file in the child-theme folder with name {filename}-template.php
And put the following in the file
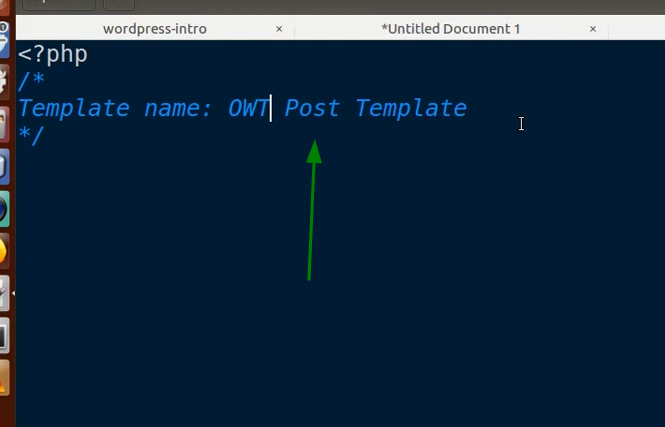
And match it with that page layout template
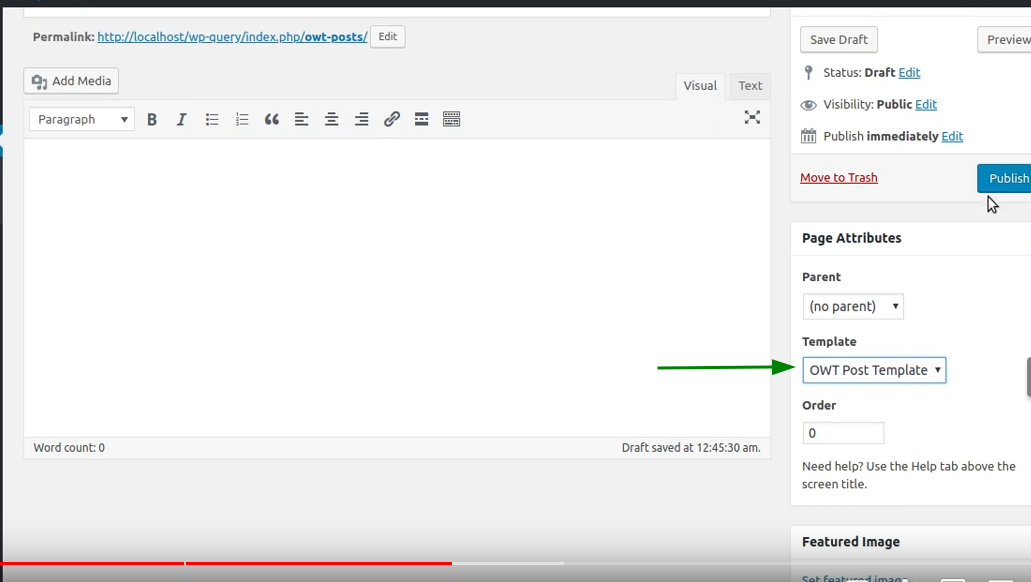
And play with WP_Query.
PS The while loop is commonly known in wordpress development as
“The loop”
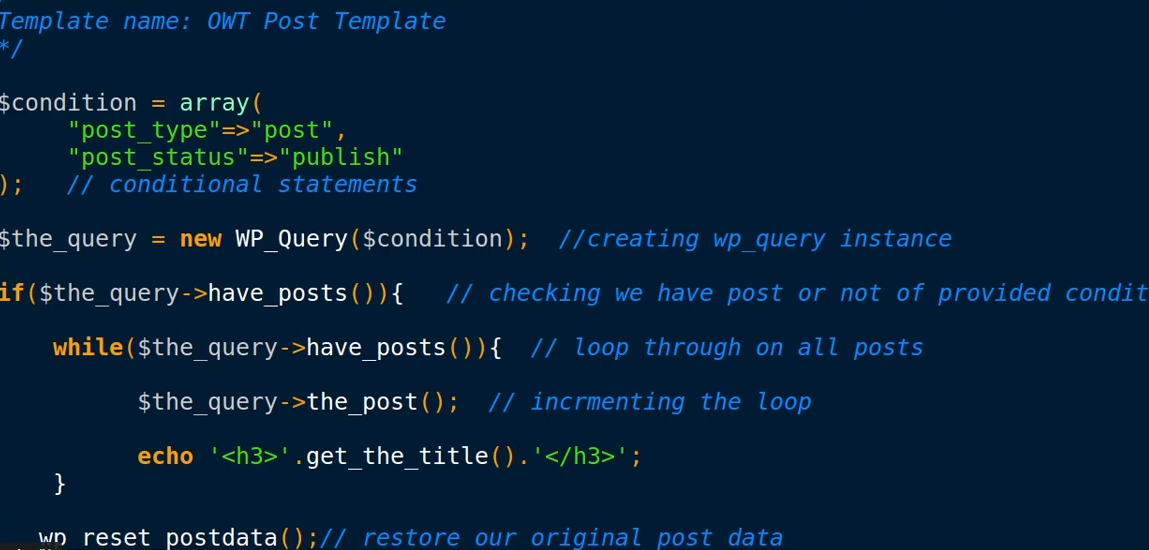
Code Example of WP_Query
<?php $args = array( 'post_type' => 'post', // Specify the post type (post, acf_name, product...) 'posts_per_page' => -1 // Number of posts to retrieve (-1 retrieves all without pagination) ); $query = new WP_Query($args); if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); //advances the current post in the loop // Do what you want to do //post_id = get_the_ID(); //Return id of current post //the_title(); //Echo the current post to the output $titelPost = get_the_title(); //Returns as a string ?> <h1><?php echo $titelPost; ?></h1> <?php //the_content(); //$contentPost = get_the_content(); //Returns the content of the post } } else { // No posts found echo ‘No posts found’; } // Restore original post data wp_reset_postdata();
<?php $args = array( 'post_type' => 'post', // Specify the post type (post, acf_name, product...) 'posts_per_page' => 5, // Number of posts to retrieve (-1 retrieves all without pagination) 'orderby' => 'post_title', // This indicates that the retrieved posts should be ordered by their titles 'order' => 'ASC' // In ascending order ); $query = new WP_Query($args); if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); //advances the current post in the loop // Do what you want to do //post_id = get_the_ID(); //Return id of current post //the_title(); //Echo the current post to the output $titelPost = get_the_title(); //Returns as a string ?> <h1><?php echo $titelPost; ?></h1> <?php //the_content(); //$contentPost = get_the_content(); //Returns the content of the post } } else { // No posts found echo ‘No posts found’; } // Restore original post data wp_reset_postdata();
<?php $args = array( 'post_type' => 'badmintonklubb', // Specifies the Custom Post Type(CPT) 'posts_per_page' => -1, // Retrieve all posts without pagination 'meta_key' => 'stats_rk', // Set the meta_key to 'stats_point'. This is the fields that a CPT have (Ordering) 'orderby' => 'meta_value_num', // Order by the numeric value of the 'stats_point' field 'order' => 'ASC', // In ascending order 'meta_query' => array( // Defines a meta query to filter posts based on custom field values array( // Third meta query condition for the boolean flag 'active_club' 'key' => 'active_club', // Check the 'active_club' field 'value' => '1', // where the value is true (1) 'compare' => '=' // Use '=' to match true ) ) ); $query = new WP_Query($args); if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); //advances the current post in the loop // Do what you want to do //$post_id = get_the_ID(); //Return id of current post //$acfFieldWins= get_field('acf_field_name', $post_id); //Returns as a string/int/checkbox/array depending acf field type $acfFieldWins= get_field('acf_field_name'); //Returns as a string/int/checkbox/array depending acf field type ?> <h1><?php echo $acfFieldWins; ?></h1> <?php } } else { // No posts found echo ‘No posts found’; } // Restore original post data wp_reset_postdata();
<?php $args = array( 'post_type' => 'product', 'posts_per_page' => -1, // Retrieve all posts without pagination 'orderby' => 'post_title', 'order' => 'DESC', ); $query = new WP_Query($args); if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); //advances the current post in the loop $titelPost = get_the_title(); //Returns as a string // Retrieve product data global $product; $title = get_the_title(); $price = $product->get_price(); $stock_amount = $product->get_stock_quantity(); } } else { // No posts found echo ‘No posts found’; } // Restore original post data wp_reset_postdata();
//get_header(); ?> <br> <h1>single-badmintonklubb.php</h1> <?php $post_id = get_the_ID(); $post = get_post($post_id); $namnBadmintonKlubb = $post->post_title; //$namnBadmintonKlubb = get_the_title($post_id); //ACF Fields $rank = get_field(‘stats_rk’); #$rank = get_field(‘stats_gp’, $post_id); $lag = get_field(‘stats_nameteam’); $antalMatcher = get_field(‘stats_gp’); //int $antalVinster = get_field(‘stats_win’); //string $antalOavgjorde = get_field(‘stats_tie’); //int $antalForluster = get_field(‘stats_loss’); //int //…. //…. //…. $pickedFruit = get_field(‘fruit’); //Select (aka combo box) $kon = get_field(‘gender’); //radio button $activeClub = get_field(‘active_club’); //Boolean true/false (but return 1 on true/checked and ” when not) // Get the field object to access its settings //$field_object = get_field_object(‘your_checkbox_field_name’); $aHealth = get_field(‘health’); //checkbox. Caution this return an array with values $bHealthSmoker = false; $bHealthFreak = false; $bHealthColdBath = false; //String representation of values in array $strChkValue = implode(“|”, $aHealth); $favClubColor = get_field(‘fav_color’); $dateFounded = get_field(‘club_founded’); //DatePicker. Try have return form in the same form as php date yyyy-mm-dd or something //Be aware of unix 1970 staring time, handling older dates can be picky…date and DateTime() // Define the delimiter $delimiter = strpos($dateFounded, ‘/’) !== false ? ‘/’ : ‘-‘; // Split the string based on the delimiter $dateComponents = explode($delimiter, $dateFounded); // Extract day, month, and year, will work YYYY/MM/DD, DD/MM/YYYY, YYYY-MM-DD and DD-MM-YYYY $firstPart = $dateComponents[0]; $secondPart = $dateComponents[1]; $thirdPart = $dateComponents[2]; if (isset($aHealth)) { foreach($aHealth as $value) { echo ” and the value is ” . $value . ” “; switch ($value) { case “healthfreak”: $bHealthFreak = true; break; case “cold_bath”: $bHealthColdBath = true; break; case “smoker”: $bHealthSmoker = true; break; } } } ?> <p>Summary</p> <h6>The id for the current post is <?php echo $post_id; ?></h6><br> <h6>The name of the klubb <?php echo $namnBadmintonKlubb; ?></h6> <h6>Klubben har antal matcher = <?php echo $antalMatcher; ?></h6> <h6>Fruit in klubb= <?php echo $pickedFruit; ?></h6> <h6>Kön hos klubb= <?php echo $kon; ?></h6> <h6>Aktiv klubb= <?php echo $activeClub; ?></h6> <!– Checkbox section –> <h5>Smoker= <?php echo $bHealthSmoker; ?></h5> <h5>Healthfreak= <?php echo $bHealthFreak; ?></h5> <h5>Cold Bath= <?php echo $bHealthColdBath; ?></h5> <h4>StringChkResult= <?php echo $strChkValue; ?></h4> <h4>DatePicker – YEAR=<?php echo $firstPart; ?> MONTH=<?php echo $secondPart; ?> DAY=<?php echo $thirdPart; ?></h4> <h4>Favorite COLOR the club has= <?php echo $favClubColor; ?></h4> <!– Skämt från partial template–> <?php get_template_part(‘_funnyjoke’); ?> <?php get_footer();
<?php //The author info is stored in the wp_user table $args = array( 'post_type' => 'post', // Specify the post type (post, acf_name, product...) 'posts_per_page' => -1, // Number of posts to retrieve (-1 retrieves all without pagination) //Filter based on author id, (table wp_user on column ID) //'author' => 2, // Filter based on author id 2 //'author' => '2,3', // Filter based on author id 2 or 3 //'author__in' => array(2,4) // Filter based author id 2 or author id 4 //'author' => -2', // Filter out(exclude) author id 2 //'author__not_in' => array(2,3) // Filter out(exclude) author id 2 or author id 3 //Filter based on nicename, (table wp_user on column user_nicename) //'author_name' => "admin2" //Filter where author is admin2 ); $query = new WP_Query($args); if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); //advances the current post in the loop // Do what you want to do //post_id = get_the_ID(); //Return id of current post //the_title(); //Echo the current post to the output $titelPost = get_the_title(); //Returns as a string ?> <h1><?php echo $titelPost; ?></h1> <?php //the_content(); //$contentPost = get_the_content(); //Returns the content of the post } } else { // No posts found echo ‘No posts found’; } // Restore original post data wp_reset_postdata();
<?php //The category info is stored in the wp_terms table $args = array( 'post_type' => 'post', // Specify the post type (post, acf_name, product...) 'posts_per_page' => -1, // Number of posts to retrieve (-1 retrieves all without pagination) //Filter based on category id, table wp_terms on columns term_id //'cat' => 3, //Filter based on categoryid(wordpress backend) 3, the id is stored in the column term_id //'cat' => "2,5", //Filter based on categoryid(wordpress backend) 2 or 5, the id is stored in the column term_id //'category__in' => array(2,6), //Filter based on categoryid(wordpress backend) 2 or 6, the id is stored in the column term_id //'cat' => -3, //Filter out (exclude) based on category id is 3, the id is stored in the column term_id //'category__not_in' => array(4,8), //Filter out (exclude) based on category id is 4 or 8, the id is stored in the column term_id //'category_name' => "fruit" // Filter based on category name, stored as a string in the column slug ); $query = new WP_Query($args); if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); //advances the current post in the loop // Do what you want to do //post_id = get_the_ID(); //Return id of current post //the_title(); //Echo the current post to the output $titelPost = get_the_title(); //Returns as a string ?> <h1><?php echo $titelPost; ?></h1> <?php //the_content(); //$contentPost = get_the_content(); //Returns the content of the post } } else { // No posts found echo ‘No posts found’; } // Restore original post data wp_reset_postdata();
<?php //The tags info is (also) stored in the wp_terms table $args = array( 'post_type' => 'post', // Specify the post type (post, acf_name, product...) 'posts_per_page' => -1 // Number of posts to retrieve (-1 retrieves all without pagination) //Filter by slug //'tag' => 'php-test-framework', //filter based on slug php-test-framework this case, stored in the column slug in table wp_terms //'tag' => 'php-test-framework,php-cake', //filter based on slug php-test-framework or php-cake this case, stored in the column slug in table wp_terms //Filter by tag id //'tag_id' => 5, //filter based on tag id this case 5, stored in the column term_id in table wp_terms //'tag__in' => array(2,5,7), //filter based on tag id this case 2 or 5 or 7. Stored in the column term_id in table wp_terms //'tag__not_in' => array(2,5,7) //Filter out(exclude) based on tag id this case 2 or 5 or 7. Stored in the column term_id in table wp_terms ); $query = new WP_Query($args); if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); //advances the current post in the loop // Do what you want to do //post_id = get_the_ID(); //Return id of current post //the_title(); //Echo the current post to the output $titelPost = get_the_title(); //Returns as a string ?> <h1><?php echo $titelPost; ?></h1> <?php //the_content(); //$contentPost = get_the_content(); //Returns the content of the post } } else { // No posts found echo ‘No posts found’; } // Restore original post data wp_reset_postdata();
<?php //The tags info is (also) stored in the wp_terms table $args = array( 'post_type' => 'post', // Specify the post type (post, acf_name, product...) 'posts_per_page' => -1 // Number of posts to retrieve (-1 retrieves all without pagination) //Filter by slug //'tag' => 'php-test-framework', //filter based on slug php-test-framework this case, stored in the column slug in table wp_terms //'tag' => 'php-test-framework,php-cake', //filter based on slug php-test-framework or php-cake this case, stored in the column slug in table wp_terms //Filter by tag id //'tag_id' => 5, //filter based on tag id this case 5, stored in the column term_id in table wp_terms //'tag__in' => array(2,5,7), //filter based on tag id this case 2 or 5 or 7. Stored in the column term_id in table wp_terms //'tag__not_in' => array(2,5,7) //Filter out(exclude) based on tag id this case 2 or 5 or 7. Stored in the column term_id in table wp_terms ); $query = new WP_Query($args); if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); //advances the current post in the loop // Do what you want to do //post_id = get_the_ID(); //Return id of current post //the_title(); //Echo the current post to the output $titelPost = get_the_title(); //Returns as a string ?> <h1><?php echo $titelPost; ?></h1> <?php //the_content(); //$contentPost = get_the_content(); //Returns the content of the post } } else { // No posts found echo ‘No posts found’; } // Restore original post data wp_reset_postdata();
<?php //The tags info is (also) stored in the wp_terms table $args = array( 'post_type' => 'post', // Specify the post type (post, acf_name, product...) 'posts_per_page' => -1 // Number of posts to retrieve (-1 retrieves all without pagination) //Filter by slug //'tag' => 'php-test-framework', //filter based on slug php-test-framework this case, stored in the column slug in table wp_terms //'tag' => 'php-test-framework,php-cake', //filter based on slug php-test-framework or php-cake this case, stored in the column slug in table wp_terms //Filter by tag id //'tag_id' => 5, //filter based on tag id this case 5, stored in the column term_id in table wp_terms //'tag__in' => array(2,5,7), //filter based on tag id this case 2 or 5 or 7. Stored in the column term_id in table wp_terms //'tag__not_in' => array(2,5,7) //Filter out(exclude) based on tag id this case 2 or 5 or 7. Stored in the column term_id in table wp_terms ); $query = new WP_Query($args); if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); //advances the current post in the loop // Do what you want to do //post_id = get_the_ID(); //Return id of current post //the_title(); //Echo the current post to the output $titelPost = get_the_title(); //Returns as a string ?> <h1><?php echo $titelPost; ?></h1> <?php //the_content(); //$contentPost = get_the_content(); //Returns the content of the post } } else { // No posts found echo ‘No posts found’; } // Restore original post data wp_reset_postdata();
FAQ
How find out the key name for a Custom Post Type
1. In the WordPress backend. Click on the tab so see all entries for that post type
The post type key/name will appear in the permalink e.i
https://examplefoo.se/wp-admin/edit.php?post_type=anonser
Mean that the key is anonser
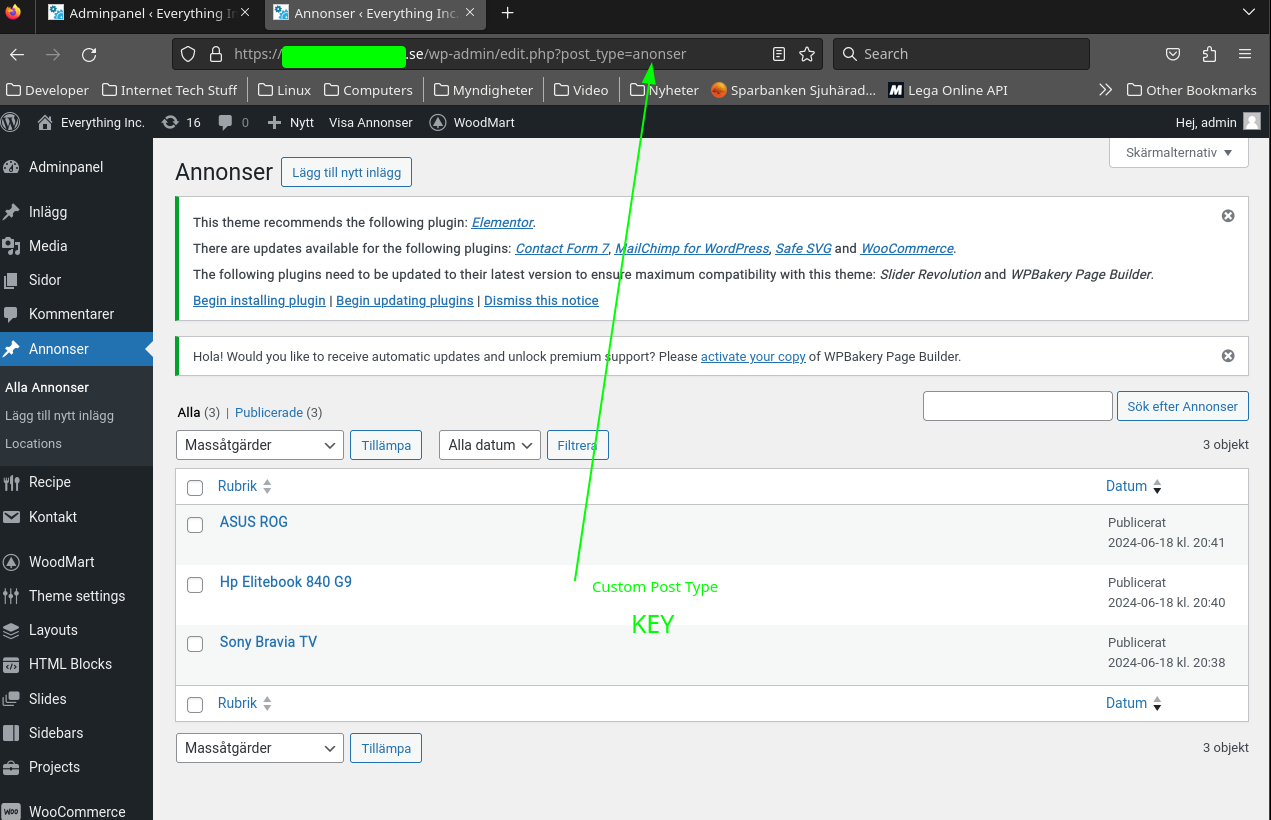
2. If using a plugin for creating the custom post type. There will be some entry there that shows the key
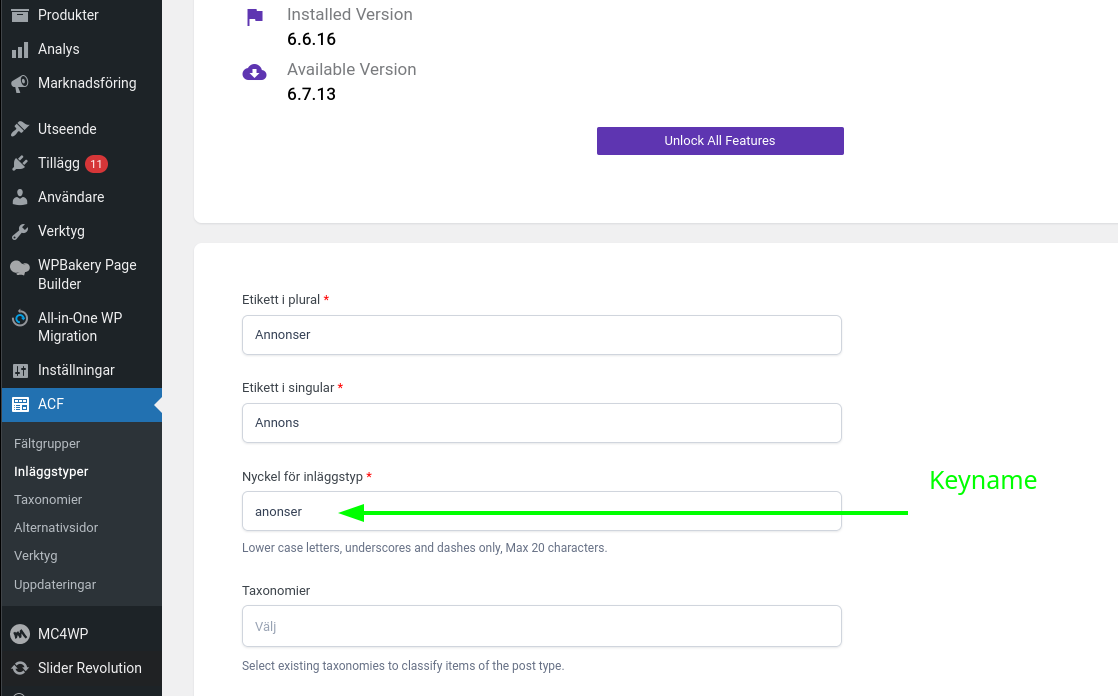
3. In code. The function that registrer a new custom post type is named
register_post_type
This function is usually found in functions.php or in a custom plugin. Common place for executing this code line is in the hook init
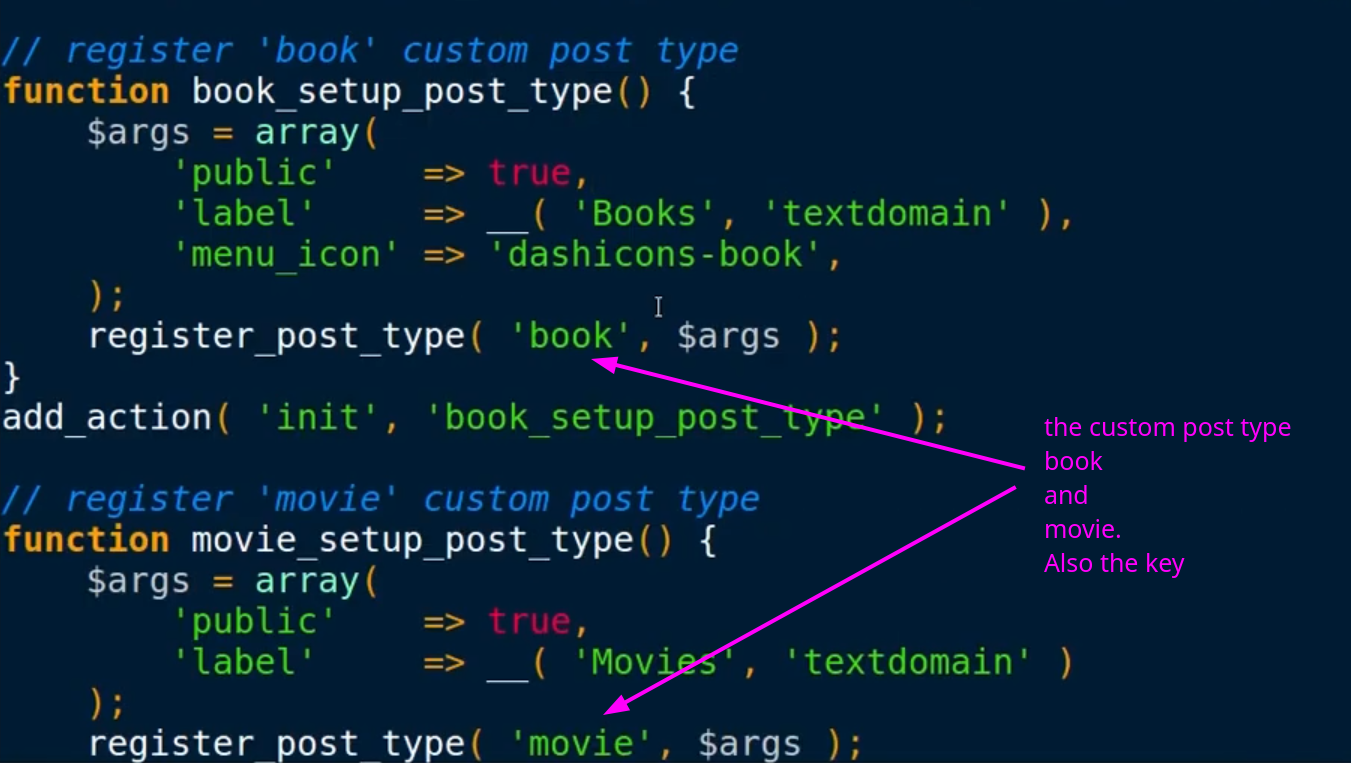