Node.js
What is Node.js
Node.js is a runtime environment that allows you to run JavaScript code outside of a web browser. It is built on the V8 JavaScript engine, which is the same engine that powers the Google Chrome browser. Node.js enables developers to write server-side code using JavaScript, traditionally a language used for client-side scripting in web browsers.
Some key features and aspects of Node.js include:
Asynchronous and Event-Driven:
Node.js uses an event-driven, non-blocking I/O model, which makes it well-suited for handling concurrent operations. This means that it can handle many connections simultaneously without getting stuck waiting for responses.Single-Threaded:
Node.js uses a single-threaded event loop architecture. While this might seem counterintuitive for handling multiple concurrent requests, Node.js is designed to efficiently delegate I/O operations to the system kernel, allowing it to handle many connections without blocking.Package Ecosystem:
Node.js has a rich ecosystem of packages available through npm (Node Package Manager), which is one of the largest package registries in the world. This allows developers to easily incorporate third-party libraries and frameworks into their projects.Cross-Platform:
Node.js is cross-platform, meaning it can run on various operating systems, including Windows, macOS, and Linux.Used for Server-Side Development:
While JavaScript was traditionally limited to client-side scripting within web browsers, Node.js allows developers to write server-side code in JavaScript. This enables full-stack development using a single language, which can streamline development processes and promote code reuse.
Node.js is commonly used to build various types of applications, including web servers, APIs (Application Programming Interfaces), real-time web applications (such as chat applications), command-line tools, and more. Its popularity has grown significantly since its introduction, and it has become a fundamental tool in modern web development. Nodejs is usually used in combination with npm package manager
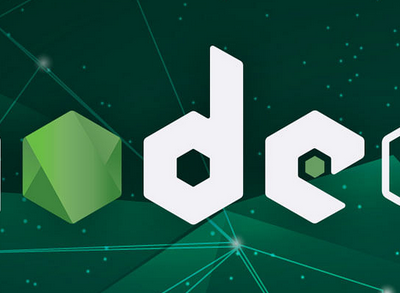
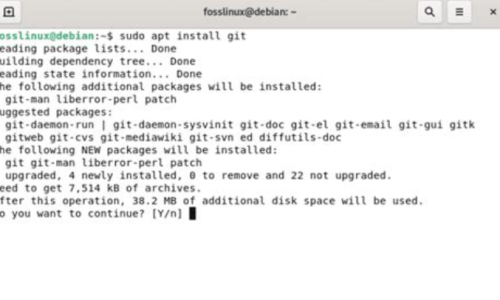
How to install node.js
Install the package
sudo apt install nodejs
Verify nodejs is installed
node -v
Install npm package (recommended
sudo apt install npm
Verify npm is installed
npm -v
Note:It possible to install node.jsnvm
also
Termomonlogy and Concept
Modules
A module in programming is a self-contained unit of code that encapsulates related functionality. Modules are used to organize code into smaller, manageable units, making it easier to develop, maintain, and reuse code.
CommonJS Modules
: Node.js uses the CommonJS module system for organizing code into reusable modules. This system allows you to define modules in separate files and then import and use them in other files using therequire()
function.javascript
-
// Example of importing a module in Node.js
const myModule = require('./myModule');
In this example,
myModule
is a module that is defined in a separate file calledmyModule.js
. Exports and Imports
: In CommonJS modules, you usemodule.exports
to export values, functions, or objects from a module, andrequire()
to import them in another module.javascript-
// main.js// myModule.js
module.exports = {
myFunction() {
console.log('This is my function');
},
myVariable: 42
};
const myModule = require(‘./myModule’);
myModule.myFunction(); // Output: This is my function
console.log(myModule.myVariable); // Output: 42 File-based Modules
: Each file in Node.js is treated as a separate module. This means that variables, functions, and other declarations in one file are not accessible in another file unless explicitly exported and imported.Core Modules
: Node.js provides a set of built-in core modules that you can use without installing them separately. These core modules offer essential functionality for tasks such as file I/O operations, networking, and cryptography.javascript-
const fs = require('fs'); // Example of importing a core module (File System)
Third-party Modules
: In addition to core modules, Node.js allows you to install and use third-party modules from the npm registry using thenpm install
command. These modules can be easily imported and used in your Node.js applications.
Core modules (node-.js built in)
Some examples of core modules in Node.js include:
fs (File System)
: Provides functions for working with the file system, allowing you to read, write, and manipulate files and directories.http
: Enables building HTTP servers and making HTTP requests. It provides classes and methods for creating servers, handling requests, and sending responses.https:
: Similar to thehttp
module but specifically for HTTPS (HTTP over SSL/TLS). It allows you to create HTTPS servers and make secure HTTPS requests.path
: Offers utilities for working with file paths and directories. It provides functions for resolving, joining, and normalizing file paths across different operating systems.os (Operating System)
: Provides information about the operating system on which Node.js is running. It includes methods for retrieving information about CPU, memory, network interfaces, and more.util
: Contains various utility functions useful for debugging, formatting, and inspecting objects. It provides functions for inheritance, type checking, and error handling.crypto
: Offers cryptographic functionality, such as encryption, decryption, hashing, and generating secure random numbers.events
: Implements the EventEmitter class, which allows you to create custom event emitters and handle events in an event-driven manner.stream
: Provides a framework for working with streams of data. Streams are used for reading from and writing to data sources asynchronously.querystring
: Offers utilities for parsing and formatting URL query strings.zlib
: Provides functions for compressing and decompressing data using zlib, a popular compression library.
These are just a few examples of core modules available in Node.js. You can find a complet
Node.js - Workflow
Workflow for Creating a node.js app
- Create a folder and cd into it
npm init
- Creates/install node_modules folder. The file package.json is created
- Create a file as the one specified as entry point in previous step
- usually javascript file
index.js
- usually javascript file
- Make a dummy js app, in index.js do e.i console.log(“Hello”);
node index.js
- Executes index.js
Adding packages with npm
- Install the packages (in this case casual) you want
npm install casual
- Use the package in code
var casual = require('casual'); //Add this code to
var city = casual.city; //to index.js
console.log(city); //Verify that it works
Commands
DESCRIPTION | COMMAND |
---|---|
Run node.js app/file |
node {filename}.js |
Enter CLI mode |
node |
Exit CLI mode |
.exit |
nodejs version |
node -v |
Display Node.js help information |
node -h node --help |
Execute JavaScript code directly from the command line |
node -e "JavaScript code" |
Start Node.js in inspector mode for debugging |
node --inspect |
Preload a module before running the script |
node --require "moduleName" |
Enable tracing of process warnings |
node --trace-warnings |
Disable printing process warnings |
node --no-warnings |
How make a node.js application that makes a woo api call
1. Create a new folder
mkdir my-node-project
2. Cd into it
cd my-node-project
3. Create the my-node-project project
npm init -y
4. Create the index.js file
touch index.js
5. Install the woocommerce rest api
npm install @woocommerce/woocommerce-rest-api
6. Insert the code into index.js. This code retrieves the order number 56
// index.js
const WooCommerceRestApi = require("@woocommerce/woocommerce-rest-api").default;
// WooCommerce API setup
const api = new WooCommerceRestApi({
url: 'https://your-woocommerce-site.com', // Replace with your WooCommerce site URL
consumerKey: 'ck_your_consumer_key', // Replace with your Consumer Key
consumerSecret: 'cs_your_consumer_secret', // Replace with your Consumer Secret
version: 'wc/v3'
});
// Function to get order information
async function getOrder(orderId) {
try {
const response = await api.get(`orders/${orderId}`);
console.log('Order Information:', response.data);
} catch (error) {
console.error('Error retrieving order:', error.response ? error.response.data : error.message);
}
}
// Retrieve information for order 56
getOrder(56);
The doc are at the page The woocommerce api doc
How make a node.js application that makes a Microsoft BC call
1. Create a new folder
mkdir my-node-bc-project
2. Cd into it
cd my-node-bc-project
3. Create the my-node-project project
npm init -y
4. Create the index.js file
touch index.js
5. Install the Odata Javascript library and basic-authorization-header module
npm install odata
npm install basic-authorization-header
6. Insert the code into index.js. (modify the pink text so it fits your requirements/need)
// index.js
const o = require('odata').o;
const basic = require('basic-authorization-header');
//base url with port number
const BC_URL = "https://nametourl.companyprovider.com:1234/companyclient/ODataV4/";
const BC_USER = 'account_name';
const BC_KEY = 'super_secret_api_token';
// Setup the handler
// Create an OData handler
const oHandler = o(BC_URL,
{
headers:
{
Authorization: basic(BC_USER, BC_KEY),
'Content-type': 'application/json;odata=verbose'
}
});
//Fetching all metadata, only base url with no query parameters
async function fetchMetaData() {
try {
const queryParams = {};
//const response = await oHandler.get().query(queryParams);
const response = await oHandler.get().query();
console.log('Response:', response);
}
catch (error) {
console.error('Error fetching endpoints items:', error);
}
}
//Fetching all products, endpoint with query parameters
async function fetchProducts() {
try {
const queryParams =
{
$filter: "pWebShop1 eq true", //Filter result
$top: 3, //Limit to 3 result elements
$select: "description,lastDateModified" //Only return these field back
};
const response = await oHandler.get('endpointpath').query(queryParams);
console.log(response);
}
catch (error) {
console.error('Error fetching products:', error);
}
}
//Fetch Metadata
fetchMetaData();
//Fetch products
fetchProducts();
require vs import
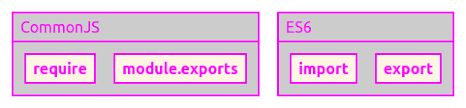
require() | import |
---|---|
Uses CommonJS syntax: require('module') |
Uses ES6 syntax: import module from 'module ' |
Used for importing modules synchronously | Used for importing modules asynchronously (dynamic) |
Supports both CommonJS modules and ES modules | Supports only ES modules (requires .mjs extension) |
No explicit support for default exports | Supports default exports (import module from 'module' ) |
Supports named exports via destructuring | Supports named exports directly (import { name } from 'module' ) |
Does not support static analysis | Supports static analysis (enables tree shaking) |
Does not supported dynamic importing | Supports dynamic importing (import() function) |
Synchronous, loads modules on demand | Asynchronous, loads modules only when needed |
Not supported in browser environments | Supported in modern browsers with module support |
require()
and import
serve the same purpose of loading modules, but import
is the newer and more feature-rich approach introduced in modern JavaScript. It’s especially useful in browser environments and when working with ES6 modules
Module exports - (CommonJS module system)
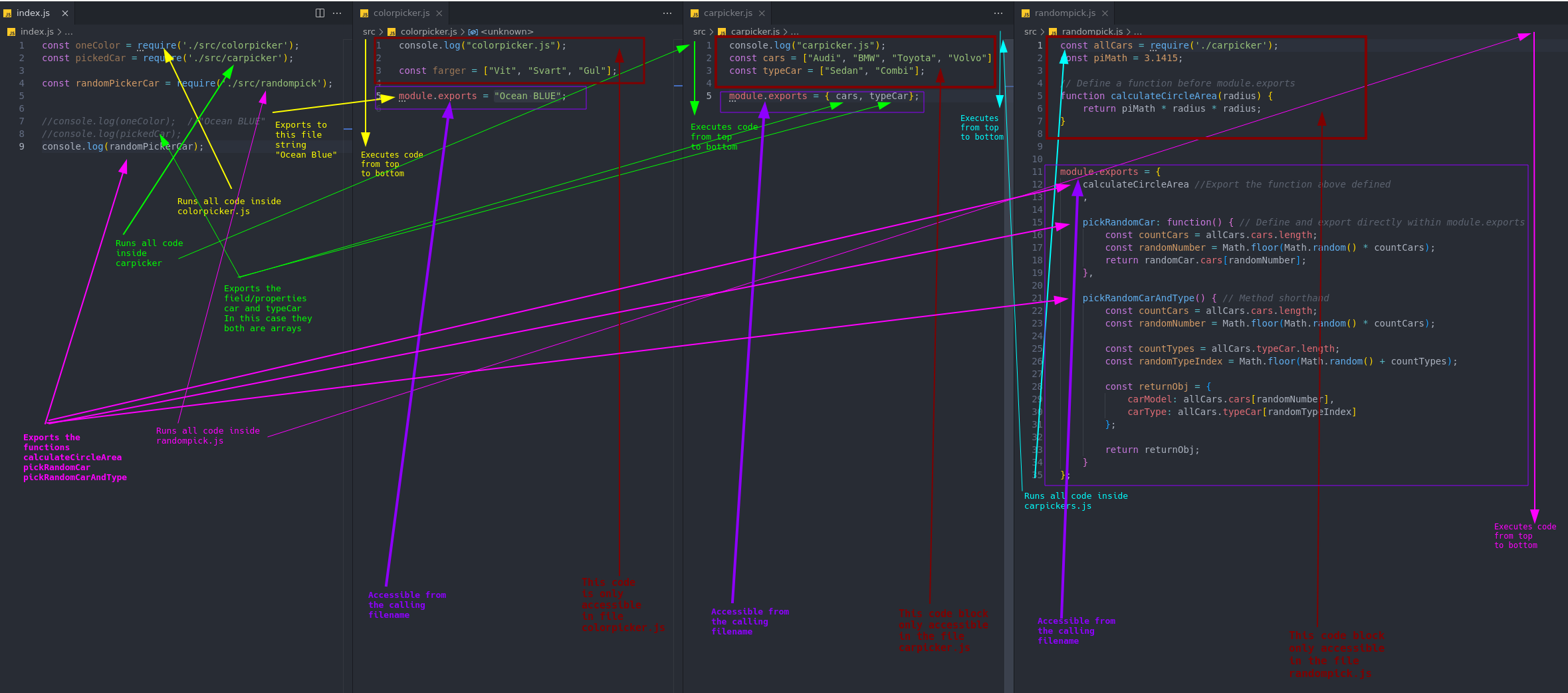
Typo, should be
pickRandomCar()
return allCars.cars[randomNumber];
pickRandomCareAndType()
const randomTypeIndex = Math.floor(Math.random() * countTypes);
Note here that both that even if multiple require is made for the same file only one time is the code executed.
E.i even multiple require('./src/carpicker')
is made only oneconsole.log(carpicker); is executed. IMPORTANT!
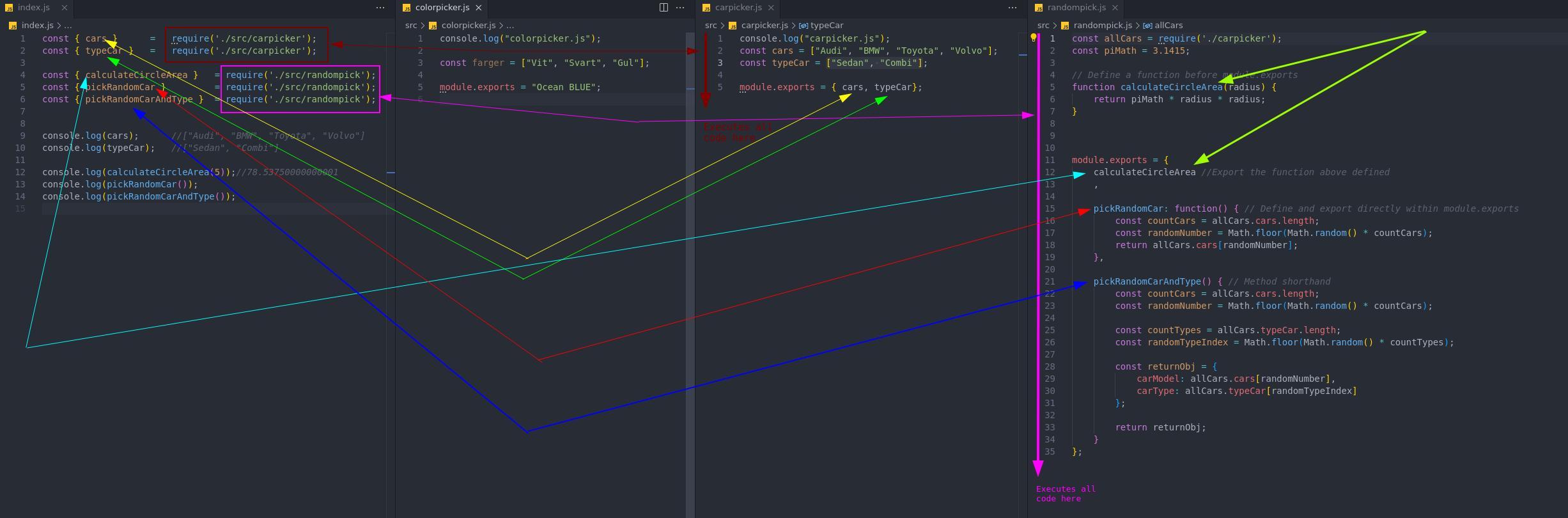
ECMA Script module - aka ESM
Before using the new ESM module system you need to set this in your package.json file
"type" : "module"
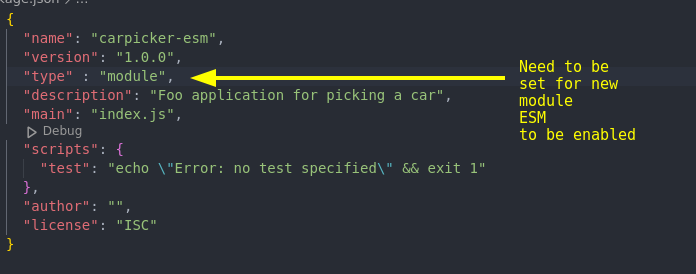
export default exports the it as a whole object
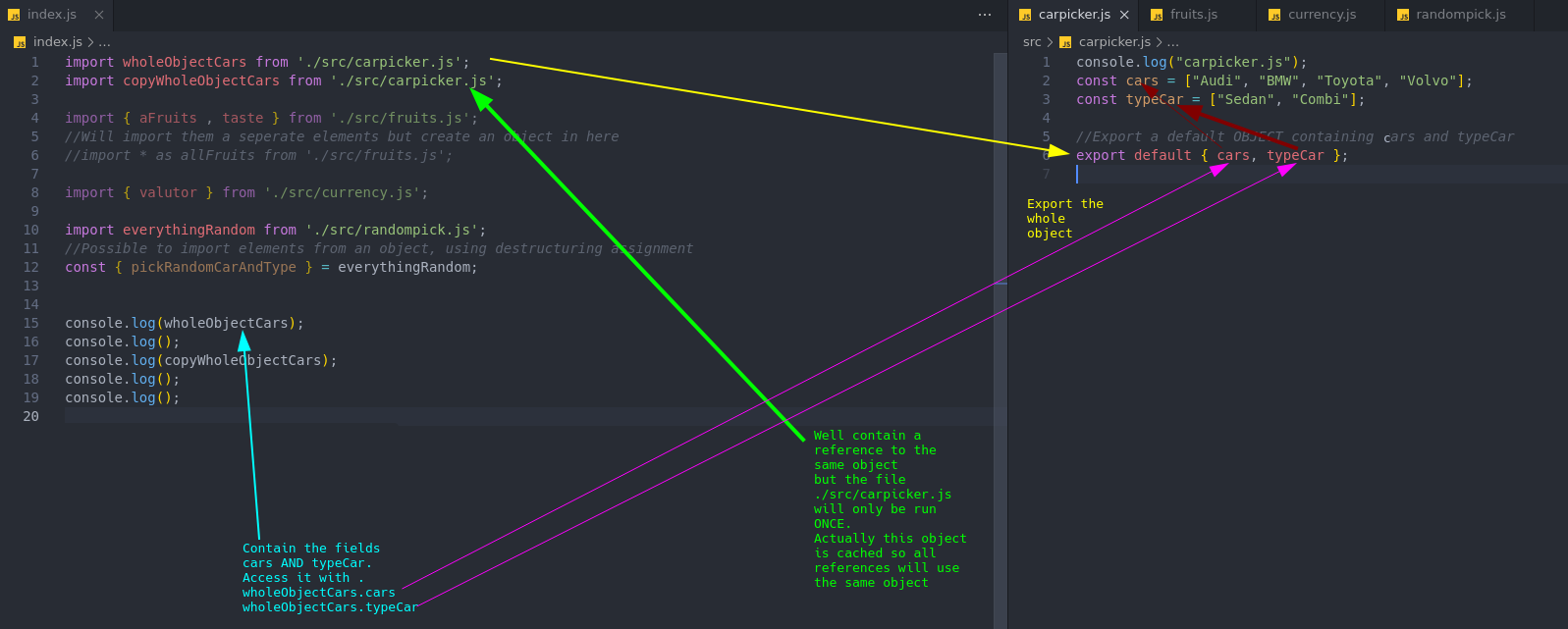
export exports the elements separately
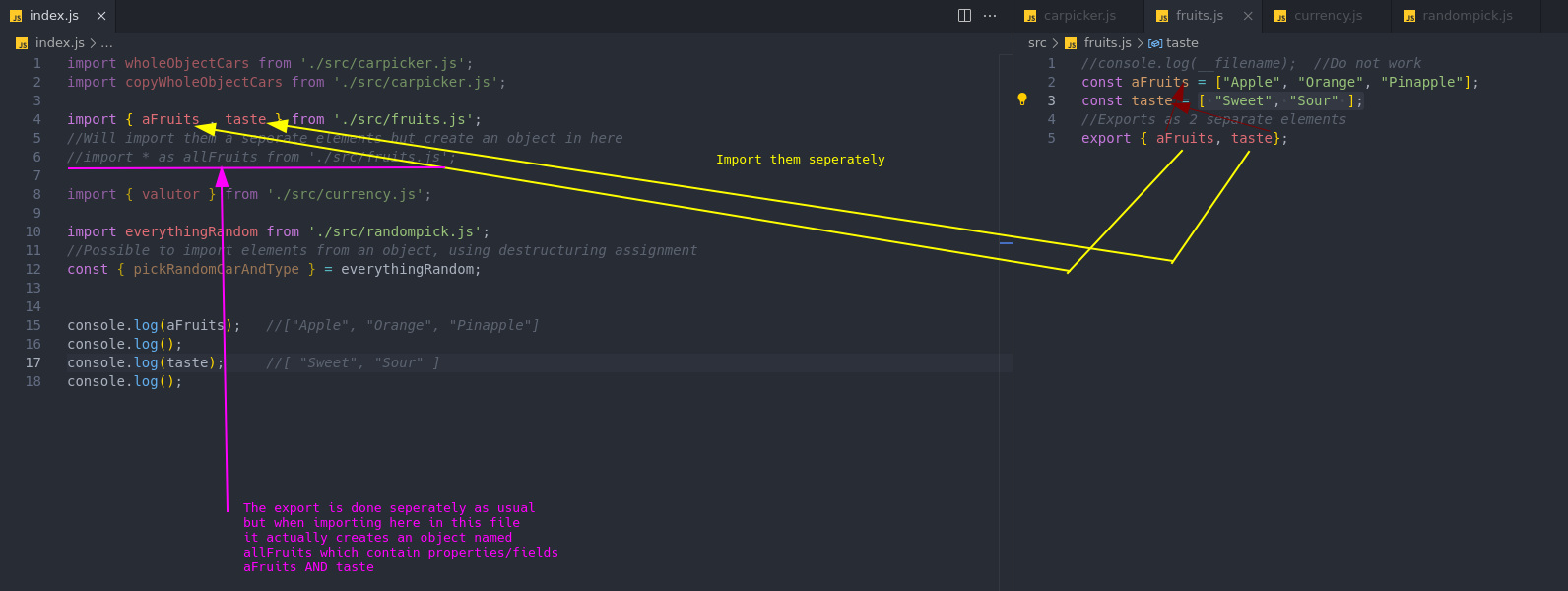
export with alias
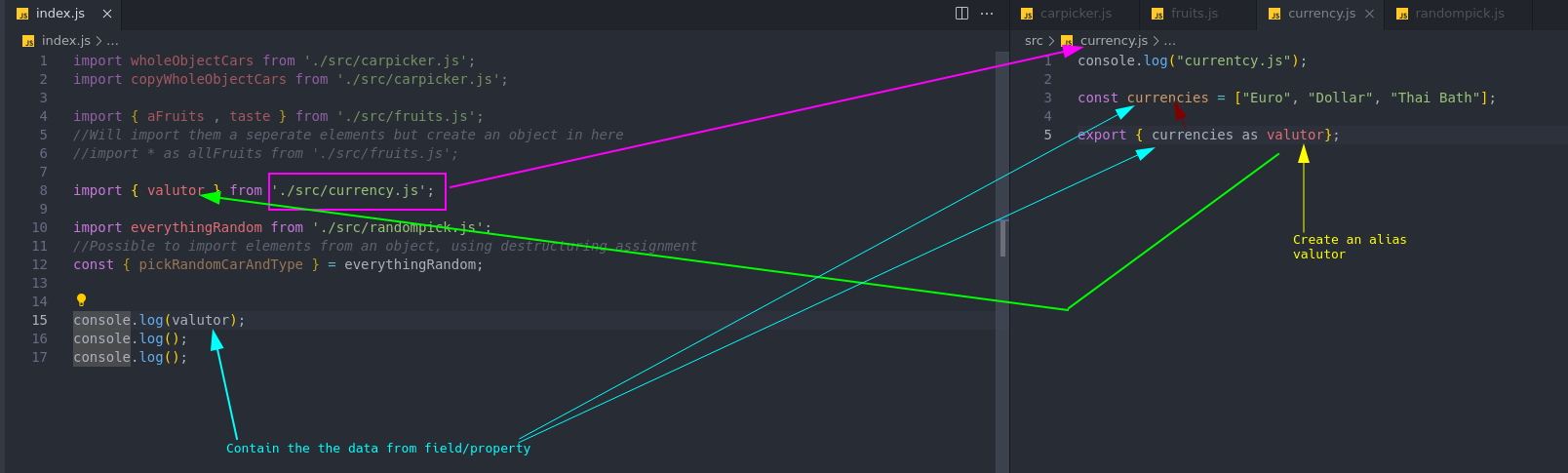
export a complex whole object
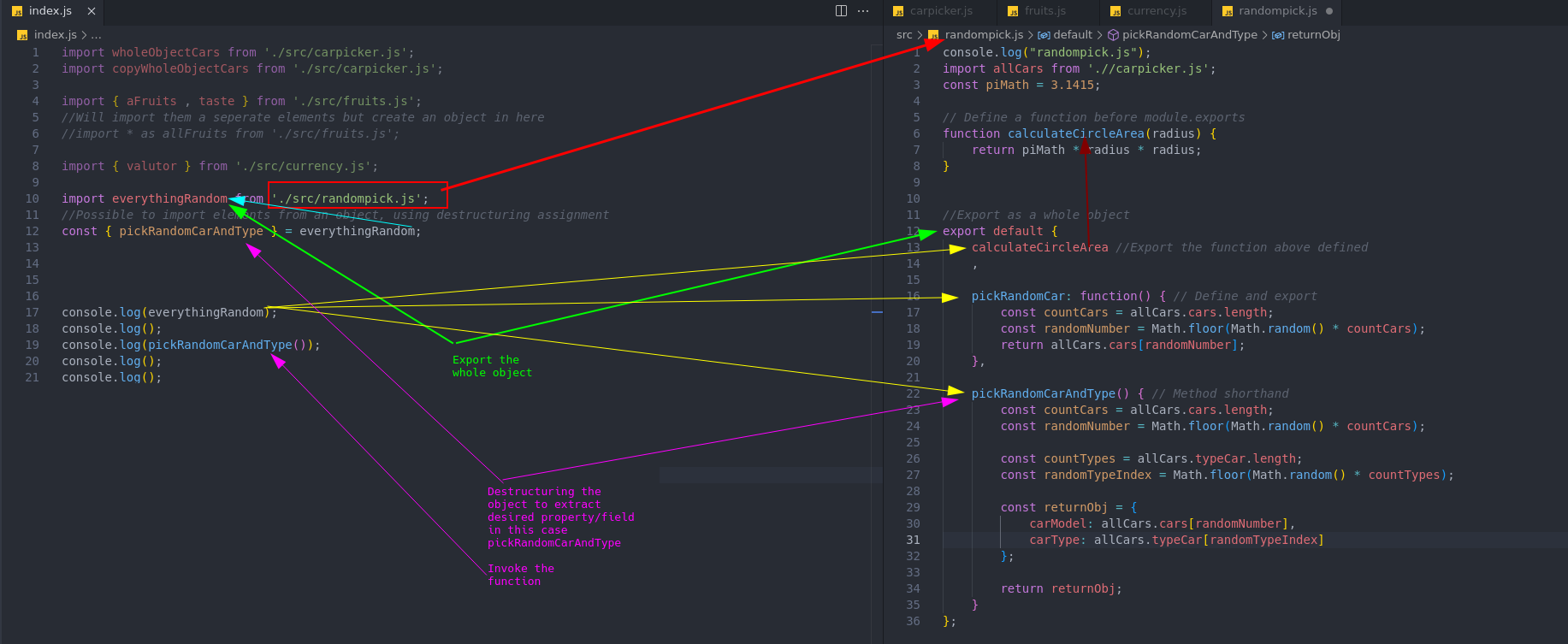
2 module systems - CoommonJS AND ES Modules (ECMAScript Modules)
CommonJS
:- This is the original module system used in Node.js.
- You define a module by using
module.exports
orexports
to export variables and functions. - You import modules using
require()
.
ES Modules (ECMAScript Modules)
:- Introduced in ECMAScript 6 (ES6) and later adopted in Node.js.
- You import modules using the
import
statement. - Requires either a
.mjs
file extension or"type": "module"
in thepackage.json
.
Key Differences
Syntax
: CommonJS usesrequire()
andmodule.exports
, while ES Modules useimport
andexport
.Loading
: CommonJS modules are loaded synchronously, while ES Modules are loaded asynchronously, allowing for better performance in certain scenarios.Compatibility
: CommonJS is primarily used in Node.js, while ES Modules are standard in modern JavaScript environments (browsers and Node.js).
Important to understand is that
both CommonJS and ES Modules serve the same fundamental purpose: to provide a way to organize and encapsulate code into reusable modules. They allow developers to:
Encapsulate Functionality
: Each module can encapsulate its own functionality, exposing only what is necessary through exports. This helps prevent naming conflicts and keeps the global scope clean.Reuse Code
: Modules can be easily reused across different parts of an application or in different projects. This promotes DRY (Don’t Repeat Yourself) principles.Organize Code
: Both systems allow developers to break down complex applications into smaller, manageable pieces, making code easier to understand and maintain.
Similarities
- Both systems enable you to import and export functionality.
- They both help structure applications in a modular way.
Differences
While they serve the same purpose, the differences in syntax, loading behavior, and compatibility can affect how you write and organize your code:
Syntax
: The syntax for importing and exporting is different.Asynchronous vs. Synchronous Loading
: ES Modules are designed to be loaded asynchronously, which can provide performance benefits, especially in web contexts.Environment
: CommonJS is widely used in Node.js environments, while ES Modules are a standard in both Node.js and browser environments.