NPM
What is NPM
NPM (Node Package Manager) is a command-line tool and an online repository of software packages or modules for Node.js, a JavaScript runtime environment. It allows developers to easily install, manage, and share reusable code packages or libraries.
With NPM, developers can:
Install Packages
: Easily add packages or libraries to their projects by running simple commands likenpm install package-name
.Manage Dependencies
: Keep track of project dependencies by specifying them in apackage.json
file. NPM handles the installation and updating of dependencies based on this file.Version Control
: Control and specify the versions of packages to be used in a project to ensure consistency and avoid compatibility issues.Publish Packages
: Developers can publish their own packages to the NPM registry, making them available for others to use.Scripts
: NPM allows developers to define custom scripts in theirpackage.json
file, enabling automation of various tasks related to building, testing, and deploying their projects.
Overall, NPM simplifies the process of managing dependencies and facilitates collaboration within the Node.js community by providing a centralized repository for sharing code.
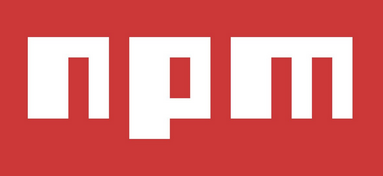
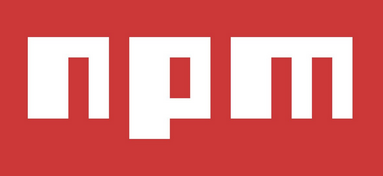
Advantages with NPM
There are several advantages to using npm:
Large Package Ecosystem
: npm hosts a vast repository of over 1.5 million packages, covering a wide range of functionalities and use cases. This extensive ecosystem allows developers to leverage existing solutions rather than reinventing the wheel, saving time and effort.Dependency Management
: npm simplifies dependency management by automatically resolving and installing dependencies for a project based on thepackage.json
file. It handles versioning, ensuring that compatible versions of packages are installed, and updates dependencies when needed.Version Control
: Developers can specify exact versions or ranges of versions for dependencies in thepackage.json
file, providing control over the packages used in a project. This helps maintain consistency across different environments and avoids unexpected changes due to updates.Ease of Use
: npm’s command-line interface (CLI) is intuitive and easy to use, allowing developers to perform common tasks such as installing packages, running scripts, and publishing packages with simple commands. This lowers the barrier to entry for developers new to package management.Community and Collaboration
: npm fosters a vibrant community of developers who share their packages and contribute to open-source projects. Developers can easily discover and reuse code from others, collaborate on projects, and contribute improvements back to the community.Custom Scripts
: npm enables developers to define custom scripts in thepackage.json
file, automating various tasks such as building, testing, linting, and deploying projects. This streamlines development workflows and improves productivity.Package Publishing
: Developers can publish their own packages to the npm registry, making them accessible to others. This encourages code sharing and facilitates collaboration within the community.
Overall, npm provides a powerful and efficient package management solution for JavaScript and Node.js projects, offering a range of features that contribute to improved productivity, code quality, and collaboration among developers.
How install npm
Install the package
sudo apt install nodejs
Verify nodejs is installed
node -v
Install npm package (recommended
sudo apt install npm
Verify npm is installed
npm -v
Termomonlogy and Concept
The package.json
file is a crucial component of Node.js projects, serving multiple purposes:
Metadata
: Thepackage.json
file contains essential metadata about the project. This metadata includes:Project Name
: The name of the project.Version
: The version number of the project.Description
: A brief description of the project.Author
: The author(s) of the project.License
: The license under which the project is distributed.Repository URL
: The URL of the project’s source code repository.
This metadata helps developers, users, and automated tools understand the purpose and characteristics of the project.
Dependency Management
: One of the most critical roles ofpackage.json
is managing dependencies. It includes:Dependencies Section
: Lists the external packages or libraries that the project depends on to function correctly.Dependency Versions
: Specifies the versions or version ranges of the dependencies required by the project.
When someone else wants to work on or run the project, they can easily install all the necessary dependencies by running a single command (
npm install
). This ensures that everyone working on the project is using the same set of dependencies, minimizing compatibility issues.Scripts
: Thepackage.json
file allows developers to define custom scripts that can be executed using thenpm run
command. This includes:Predefined Scripts
: Scripts for common tasks such as building the project, running tests, linting code, starting a development server, etc.Custom Scripts
: Developers can define their own scripts tailored to specific project requirements.
These scripts streamline common development tasks, improve workflow efficiency, and ensure consistency across development environments.
Project Configuration
:package.json
can include configuration settings for various tools and libraries used in the project. This includes:Tool Configuration
: Settings for build tools, compilers, bundlers, test runners, linters, etc.Environment Configuration
: Environment-specific settings for development, testing, and production environments.Deployment Configuration
: Configuration for deployment automation tools and platforms.
By centralizing project configuration in
package.json
, developers can easily manage and share project settings, ensuring consistent project behavior across different environments.
Overall, the package.json
file serves as a comprehensive configuration and management file for Node.js projects, providing metadata, managing dependencies, defining scripts, and facilitating project management and collaboration.
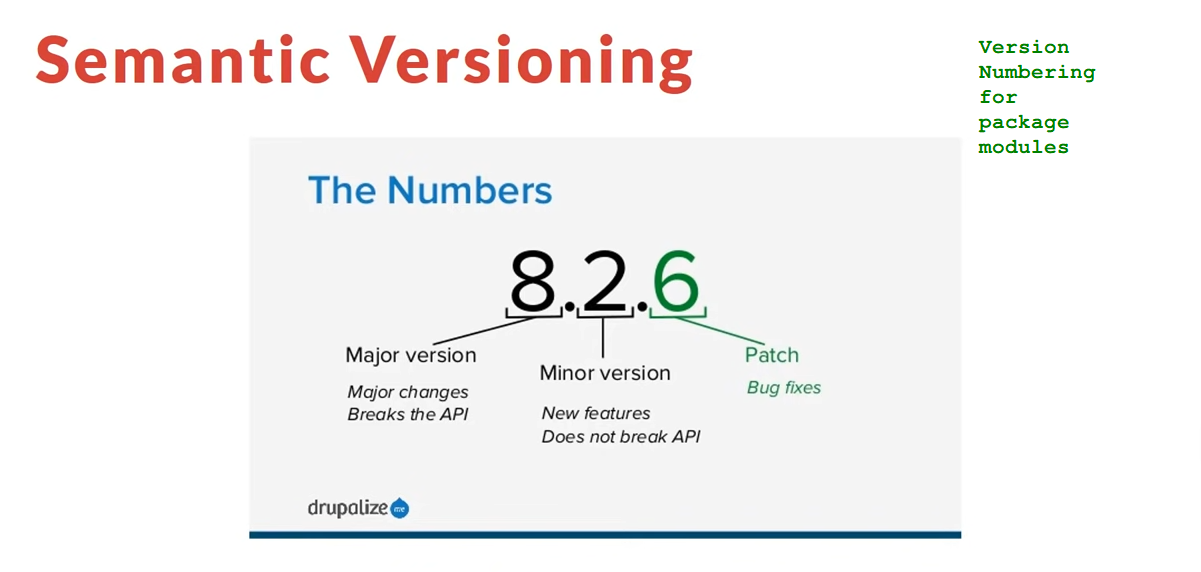
NPM - Workflow
When you install a package locally, npm will add it to the dependencies
section of the package.json
file in your project directory. If you want to add a package as a development dependency (only needed for development purposes, not in production), you can use the --save-dev
flag:
clearing the npm cache is usually a safe operation
Commands
DESCRIPTION | COMMAND |
---|---|
This command creates the file package.json |
npm init |
Installes all packages that are in the package.json file |
npm install |
Installs the package globally (system-wide) |
npm install -g {package-name} |
Installs the package locally |
npm install {package-name} |
Install package as a development dependency |
npm install --save-dev {package-name} |
Uninstalls a package and removes it from the dependencies or devDependencies section of package.json. |
npm uninstall {package-name} |
Updates installed packages to their latest versions based on the versioning rules specified in package.json.. |
npm update |
Checks for outdated packages in the current project |
npm outdated |
Lists installed packages and their dependencies |
npm list |
Searches for packages on the npm registry |
npm search {keyword} |
Executes a custom script defined in the scripts section of package.json. |
npm run {script-name} |
npm looks for a script named "start" in the scripts section of the package.json file. It then executes the command specified as the value of the "start" script. |
npm start |
Manages npm configuration settings.. |
npm config |
NPM version |
npm -v |
npm will delete all the contents of the cache |
npm cache clean |
Cleans the entire npm cache forcibly |
npm cache clean --force |
Cleans all the cached data, including the package data and metadata. |
npm cache clean --all |
Performs a dry run, showing what would be deleted without actually deleting anything |
npm cache clean --dry-run |
Cleans the cache for a specific package only |
npm cache clean {package-name} |
Command is used to remove "extraneous" packages from the node_modules directory. Extraneous packages are those that are not listed as dependencies in the package.json file |
npm prune |