NVM
What is NVM
In a Node.js environment, NVM stands for Node Version Manager. It’s a tool that allows you to install, manage, and switch between different versions of Node.js on your system.
With NVM, you can:
- Install multiple versions of Node.js.
- Easily switch between these versions.
- Set a default Node.js version for new shell sessions.
- Test your code across different Node.js versions.
This is especially useful for developers who need to maintain different projects that depend on different Node.js versions. One thing to note that when using nvm it’s best to NOT actually install nodejs, because nvm will handle this
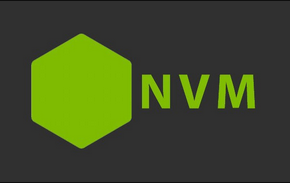
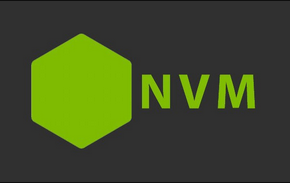
How to install nvm
Note if you have a nodejs installed already it's preferable to uninstall it BEFORE installing nvm
1) Download and install dependencies
apt install build-essential libssl-dev
1a) Download with curl
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/master/install.sh | bash
OR
1b) Download with wget
wget -qO- https://raw.githubusercontent.com/nvm-sh/nvm/master/install.sh | bash
and it will execute the script with bash!
2 Source the file
. ~/.nmv/nvm.sh
DONE installing!
3 Verify nvm is installed. This will return a version number
nvm -v
4. Determine which node is on the path/active
which node
5. Install a node version(will also install npm)
nvm install 18.20.4
Note – Each node version will have it own npm version
Termomonlogy and Concept
Here are some of the most useful terminology when working with NVM:
Node.js
- JavaScript runtime that NVM manages.
Version
- Specific releases of Node.js (e.g.,
v14.17.0
), managed by NVM.
- Specific releases of Node.js (e.g.,
Install
- Command to download and set up a specific version of Node.js.
- Example:
nvm install 16.0.0
.
Use
- Switch between different Node.js versions.
- Example:
nvm use 14.0.0
.
List (ls)
- Shows all installed Node.js versions.
- Example:
nvm ls
.
LTS (Long Term Support)
- Stable versions of Node.js with long-term maintenance.
- Example:
nvm install --lts
.
nvm alias
- Create aliases for versions (e.g.,
nvm alias default 14.0.0
sets version 14.0.0 as default).
- Create aliases for versions (e.g.,
nvm current
- Displays the currently active Node.js version.
Global Packages
- NPM packages installed globally are tied to the specific Node.js version in use.
.nvmrc
- A file in your project that specifies which Node.js version to use. Running nvm use in a directory with .nvmrc will switch to the version specified in the file.
default nvm
- The default NVM version refers to the version of Node.js that NVM automatically selects and uses whenever you open a new terminal or shell session, unless you explicitly specify a different version.
nvm - Workflow
Here’s the NVM workflow using a list format:
- Install NVM
- Install NVM from the official NVM repository.Se start of this page
- Install a Node.js Version
- Install a specific version of Node.js:
- nvm install <version>
- Example:
nvm install 16.0.0
.
- Install a specific version of Node.js:
- Switch Node.js Version
- Switch to a specific version:
- nvm use <version>
- Example:
nvm use 14.17.0
.
- Switch to a specific version:
- Set a Default Node.js Version
- Set a default Node.js version for all future terminal sessions:
- nvm alias default <version>
- Example:
nvm alias default 14.0.0
.
- Set a default Node.js version for all future terminal sessions:
- List Installed Node.js Versions
- View all Node.js versions installed on your system:
- nvm ls
- View all Node.js versions installed on your system:
- View Available Node.js Versions
- See all available Node.js versions for installation:
- nvm ls-remote
- See all available Node.js versions for installation:
- Run Script with Specific Node.js Version
- Run a script with a specific Node.js version without switching:
- nvm exec <version> node <script.js>
- Example:
nvm exec 12.0.0 node app.js
.
- Run a script with a specific Node.js version without switching:
- Create
.nvmrc
File- Create a
.nvmrc
file in your project with the required Node.js version:- echo “14.17.0” > .nvmrc
- Use the version specified in
.nvmrc
:- nvm use
- Create a
- Install Global Packages for Specific Node.js Version
- After switching to a version, install global packages for that version:
- npm install -g <package>
- After switching to a version, install global packages for that version:
- Uninstall a Node.js Version
- Remove a specific version of Node.js:
- nvm uninstall <version>
- Example:
nvm uninstall 12.0.0
.
- Remove a specific version of Node.js:
This list-based workflow helps streamline the process of managing Node.js versions using NVM.
nvm workflow - Create .nvmrc file
1. Create file .nvmrc i project base/root folder
touch .nvmrc
2a/ Enter node version
echo "v18.19.0" > .nvmrc
or
2b/
node -v > .nvmrc
Commands
DESCRIPTION | COMMAND |
---|---|
Returns version of nvm | nvm -v |
List all version currently installed and latest LTS version(s) |
nvm ls |
List all available node version that could be installed |
nvm ls-remote |
Return path to currently node version in use | which node |
Install node version 14 (fermium) Note Installs the latest of version 14 |
nvm install 14 |
Install specific node version In this case 16.20.2 |
nvm install 16.20.2 |
Uninstall a specific node version In this case 20.4.0 |
nvm uninstall 20.4.0 |
Switch to another installed node version NOTE will ALSO change npm version |
nvm use "version" |
Switch to node version 16 (latest) NOTE will ALSO change npm version |
nvm use 16 |
Switch to node version specified in file .nvmrc That file needs to be in the node root/base project folder and need only contain a version number Useful when many project have different node version to toggle |
nvm use |
Change the default version | nvm alias default "version" |
Change the default version to latest 18 |
nvm alias default 18 |