AJAX
What is Ajax
AJAX, or Asynchronous JavaScript and XML, is a technique used in web development that allows for the asynchronous exchange of data between a client (usually a web browser) and a server without requiring a full page refresh. This means that web applications can retrieve data from a server in the background while the user continues to interact with the page. AJAX primarily uses JavaScript to make HTTP requests to the server, which can then respond with data, typically in formats such as JSON or XML. This enhances user experience by making web applications feel faster and more responsive, as updates can occur dynamically without disrupting the user’s workflow.
The core of AJAX functionality relies on the XMLHttpRequest
object, which is responsible for handling requests and responses between the client and server. With the advent of newer APIs like the Fetch API, the syntax for making asynchronous requests has become more streamlined and user-friendly. AJAX can be used for a variety of tasks, including loading new content, submitting form data, and retrieving data from APIs. By reducing the need for full page reloads, AJAX contributes significantly to the development of Single Page Applications (SPAs), where user interactions lead to dynamic content updates rather than traditional navigation.
In summary, AJAX programming represents a fundamental shift in how web applications communicate with servers, paving the way for more interactive and fluid user experiences. By leveraging asynchronous data fetching, developers can create applications that are not only faster but also more efficient, allowing for seamless interaction without the delays associated with full page reloads. As web technologies continue to evolve, AJAX remains a crucial component in modern web development, enabling the creation of rich, interactive user interfaces.
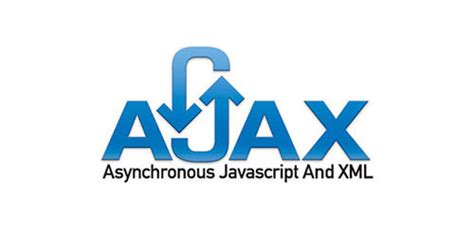
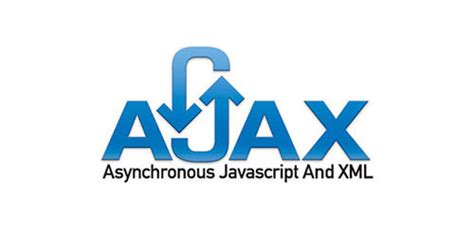
God to know about ajax
es, when you are using AJAX (Asynchronous JavaScript and XML), there must be a server involved because AJAX is primarily used to communicate with a server asynchronously. Here’s how it works and why a server is required:
Why is a Server Required?
AJAX Purpose
:- AJAX is used to send requests to a server in the background, without refreshing the entire webpage. The purpose is to fetch or send data (e.g., to/from a database or a file) dynamically from a server, and then update parts of the webpage based on the response.
Client-Server Communication
:- When an AJAX request is made, it’s either a GET or POST request (or any other HTTP method) to a server. The server processes the request, performs some action (like retrieving data, performing a database query, or executing logic in PHP, Node.js, etc.), and sends a response back to the client (browser).
Server-Side Languages
:- The server often runs a language like PHP, Node.js, Python, or Ruby, which can handle HTTP requests, execute logic, interact with a database, and return the response to the client.
AJAX Flow
Client-side (Browser)
:- The browser (via JavaScript/jQuery) makes an AJAX request, usually for fetching or sending data. This could be a request for a JSON file, HTML content, or any other data.
Server-side
:- The server receives this request, processes it (for example, by querying a database or reading a file), and returns the appropriate response (such as JSON data or HTML) back to the browser.
Response Handling (Browser)
:- The client (browser) receives the response and updates parts of the webpage dynamically, without reloading the entire page.
jquery
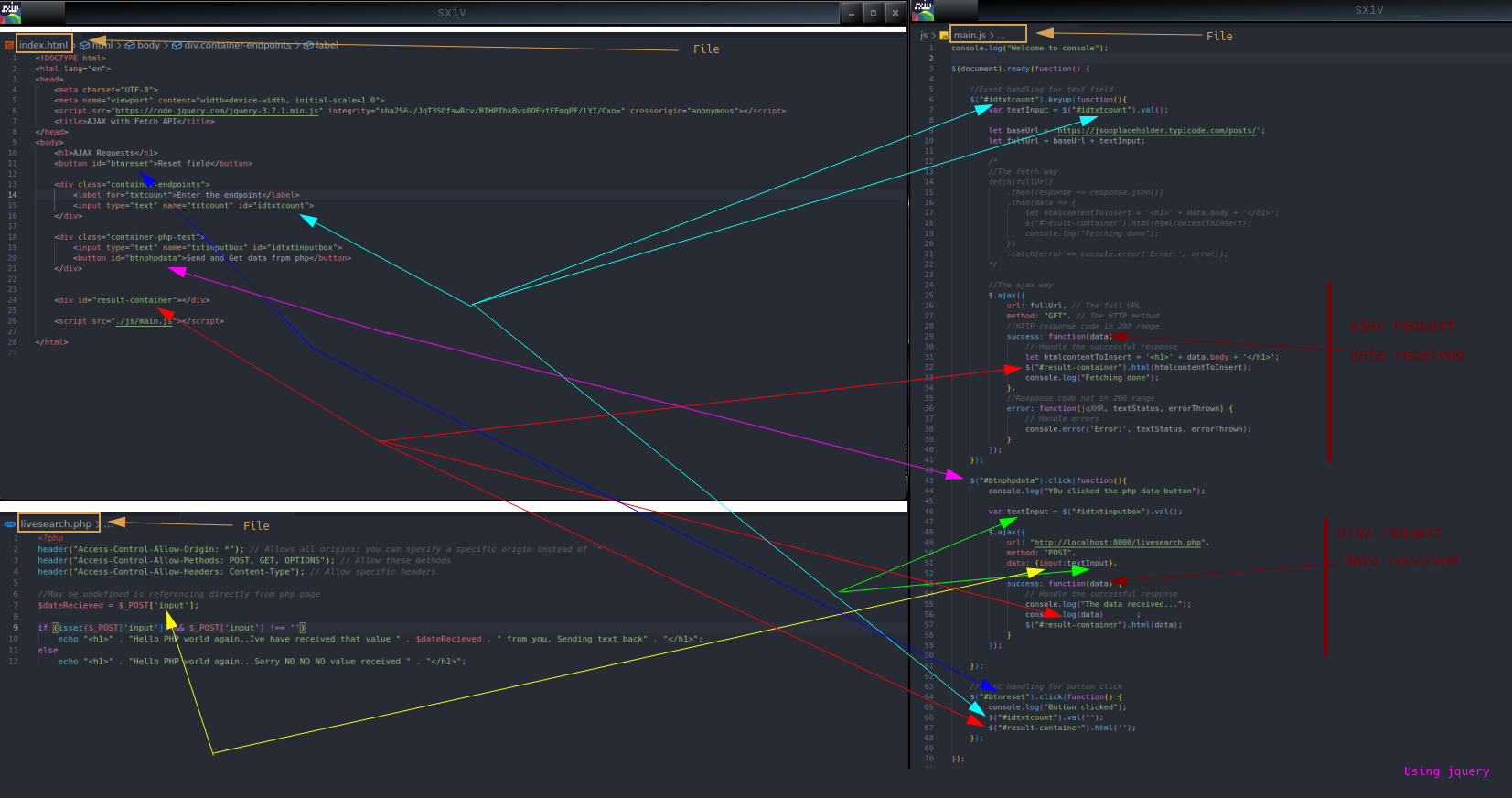
in main.js
when data is sent to php file, livesearch.php
it is the value of the variable textInput
. You later extract the value through the key input
inside the livesearch.php
Note 1: You access these key throuhg php superglobals, e.i $_GET, $_POST and so on
Note 2: the key input is actually set/defined in $_POST[‘input’] so check for an empty string
The function success and error is called (event handler. The parameter(named data in this instance) received in the function contains the entire output. In this case, everything that is echo:ed
Ajax Live Search - Mysql
index.html
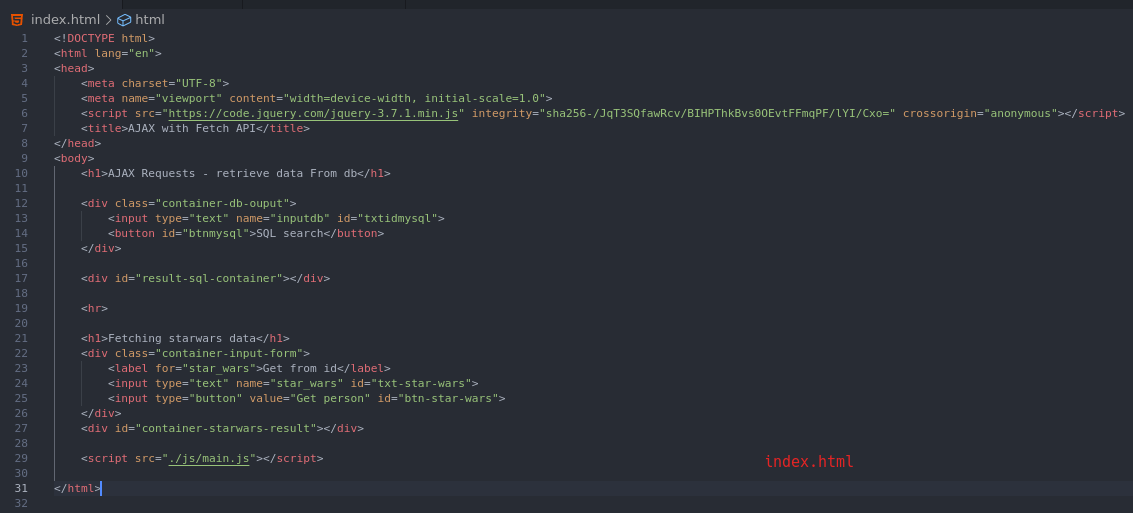
main.js
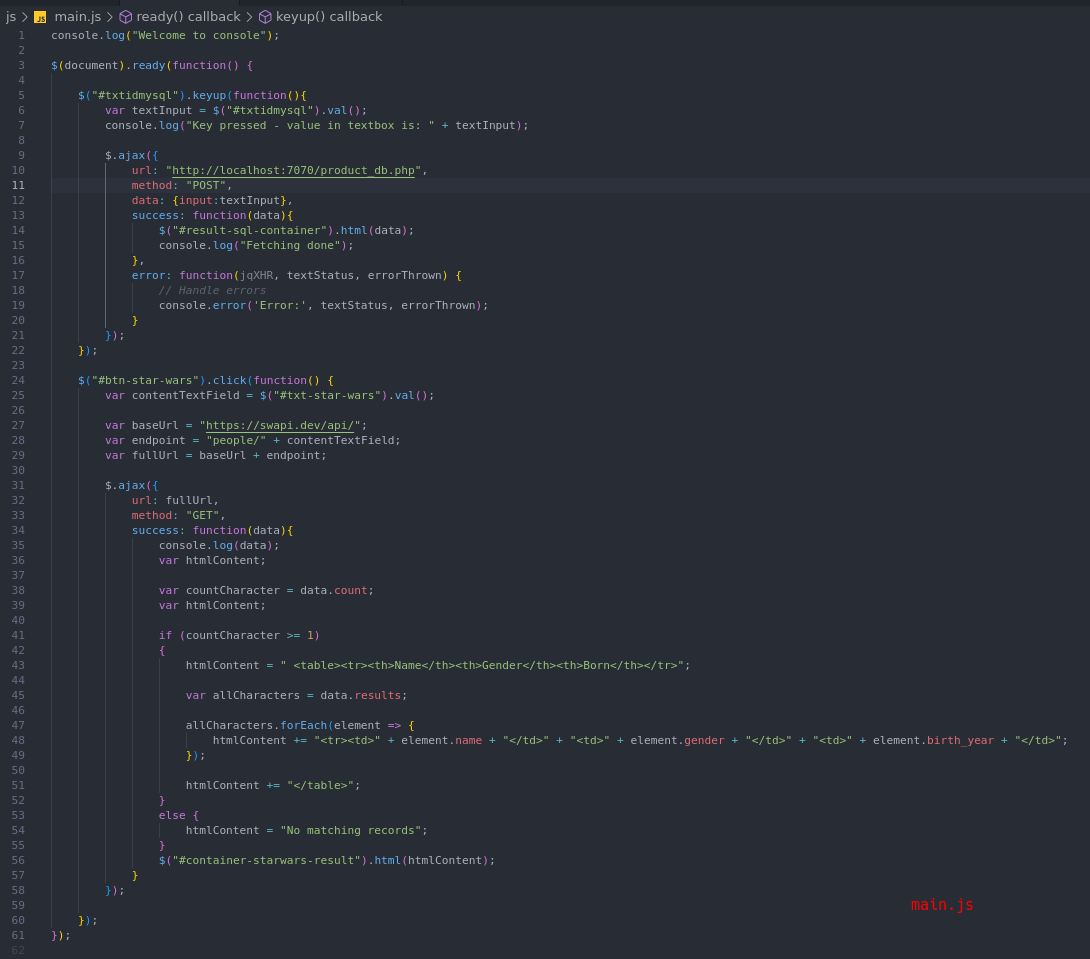
products_db.php
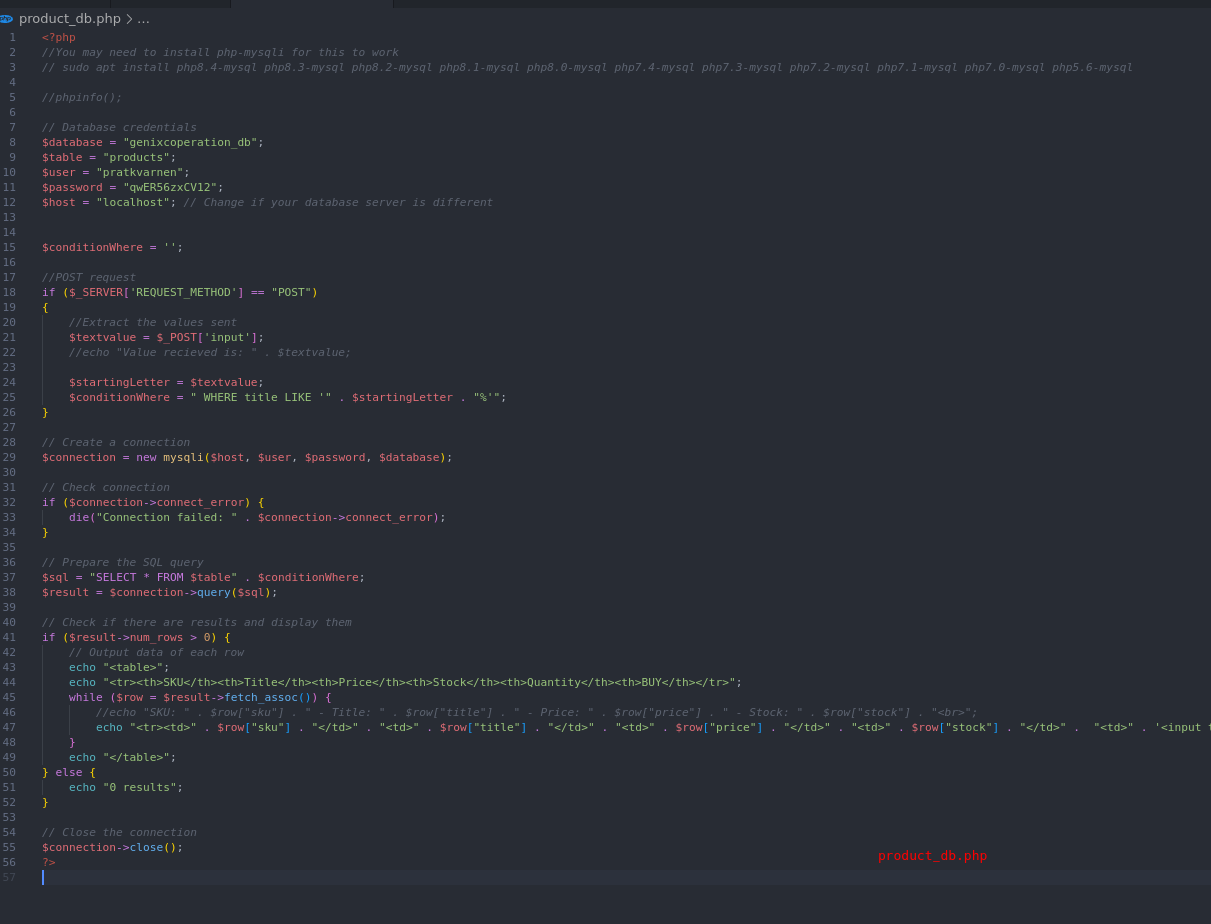
Raw html
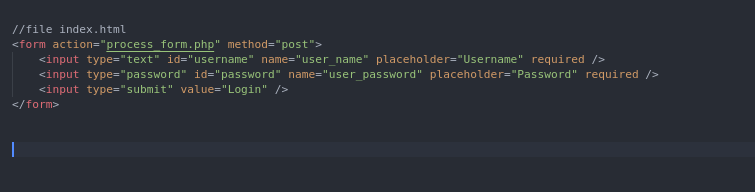
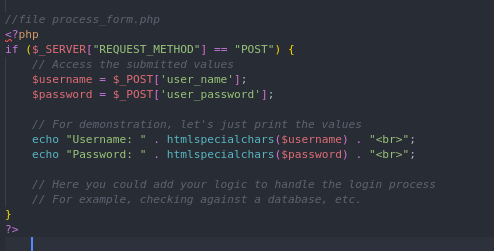