functions.php
What is functions.php
The WordPress functions.php file is a theme file that you can use to add custom code snippets to your site. You can use these code snippets to modify how different areas of your site function or add new content/code to your site.Despite being included in your theme, the WordPress functions.php file is not limited to just making customizations to your theme.
The file is called EVERY time a page is requested for the active theme .Its the most common place to add actions and filters (hooks).
Beware that additionally caching mechanisms can affect how often the functions.php
file is called.
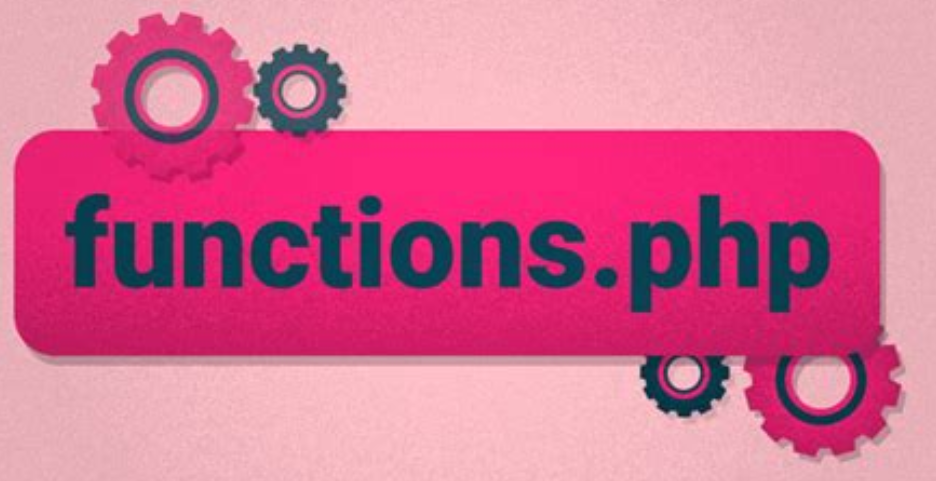
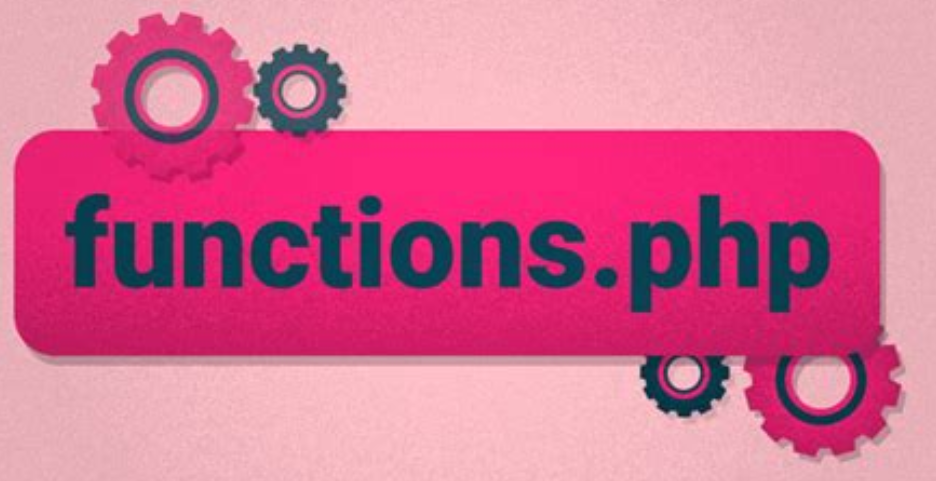
How to use functions.php
The file is located at the folder of the active theme or child-theme, /wp-content/themes/[active-theme-name]/functions.php
Termomonlogy and Concept
- Hooks: Actions and filters that allow developers to modify WordPress functionality. Functions in the
functions.php
file are often hooked into these to add or change features. - Actions: Functions that are triggered at specific points during the WordPress execution process, such as
init
,wp_head
, orwp_footer
. - Filters: Functions that modify specific data before it’s processed or displayed, such as modifying the content of a post before it’s shown on the front-end.
- Enqueueing Scripts and Styles: The process of adding CSS stylesheets and JavaScript files to the WordPress site using functions like
wp_enqueue_style
andwp_enqueue_script
. - Theme Customization: The use of the WordPress Customizer API to allow users to customize various aspects of the theme, often implemented through the
functions.php
file. - Child Themes: A theme that inherits the functionality and styling of another theme, known as the parent theme. Child themes often use the
functions.php
file to override or extend the functionality of the parent theme. - Theme Activation: Actions and functions that are executed when a theme is activated, commonly used in the
functions.php
file to set default options or perform setup tasks. - Custom Functions: PHP functions created specifically for the theme, which are added to the
functions.php
file to extend WordPress functionality or provide theme-specific features. - Error Handling: Techniques for handling errors and debugging information in the
functions.php
file, such as usingerror_log
orvar_dump
for troubleshooting. - Security: Best practices for securing the
functions.php
file and preventing unauthorized access or malicious code injection, including proper file permissions and validation of user input.
Advantage vs Disadvantage
Advantages:
- Theme-specific Customization: Allows for theme-specific customization without modifying core WordPress files.
- Centralized Location: Provides a centralized location for adding custom functions, hooks, and filters specific to the theme.
- Integration with Child Themes: Facilitates the creation and customization of child themes by overriding or extending the functionality of the parent theme.
- Seamless Theme Updates: Customizations made in the
functions.php
file are retained even after updating the theme, ensuring compatibility and consistency. - Flexibility and Extensibility: Enables developers to extend WordPress functionality by adding custom PHP functions and modifying theme behavior using hooks and filters.
- Performance Optimization: Allows for efficient loading of scripts and stylesheets by enqueueing them selectively based on the theme’s requirements.
- Debugging and Error Handling: Provides a platform for debugging code and handling errors, helping developers troubleshoot issues effectively during theme development.
Disadvantages:
- Potential for Theme Lock-in: Customizations made in the
functions.php
file are tied to the theme, making it challenging to switch themes without losing functionality. - Complexity and Maintenance: As the
functions.php
file accumulates custom code, it can become complex and difficult to maintain, especially for large-scale themes. - Risk of Theme Breakage: Incorrectly implemented customizations or conflicting code in the
functions.php
file can break the theme or lead to unexpected behavior. - Limited Scope: Customizations made in the
functions.php
file are limited to the theme’s functionality and cannot be accessed globally across multiple themes or plugins. - Security Concerns: Poorly coded custom functions or vulnerabilities in the
functions.php
file can pose security risks, potentially leading to unauthorized access or malicious attacks. - Dependency on Theme: Custom functionality added via the
functions.php
file relies on the active theme, limiting its portability and reusability across different WordPress installations or projects. - Debugging Challenges: Debugging issues in the
functions.php
file can be challenging, especially when dealing with complex interactions between custom code and WordPress core functionality.
Basic init Content in functions.php
<?php
/**
* Enqueue script and styles for child theme
*/
function woodmart_child_enqueue_styles()
{
wp_enqueue_style(‘child-style’, get_stylesheet_directory_uri() . ‘/style.css’, array( ‘woodmart-style’ ), woodmart_get_theme_info(‘Version’));
}
add_action(‘wp_enqueue_scripts’, ‘woodmart_child_enqueue_styles’, 10010);
#Test shortcode
add_shortcode(‘visabjorn’, ‘display_bjorn_fn’);
function display_bjorn_fn()
{
return “Red PILL2”;
}