Hooks
What is a hook
A WordPress hook is a point in the WordPress codebase where developers can add their own custom code to modify or extend the functionality of WordPress themes or plugins. Hooks are categorized into two types: action hooks, which allow you to execute custom code at specific points in WordPress actions, and filter hooks, which enable you to modify data or content before it is displayed on the website.
Generic term in WP that allow your plugin to hook into the rest of the WordPress, by calling YOUR custom function at a specific time. Hooks are the foundation of WordPress plugin and theme development. Enables working with custom hooks from third-party developers
Each library or framework may have its own set of hooks, events, or extension points that developers can leverage to customize or extend its functionality.
Hooks themselves do NOT
modify the underlying data directly. Instead, they provide a mechanism for developers to intercept, manipulate, or extend the data before it’s used or displayed.
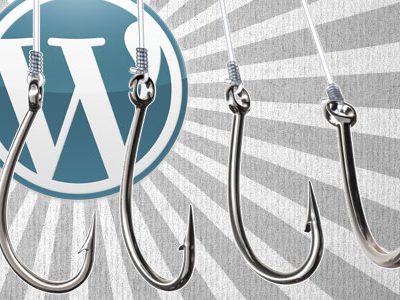
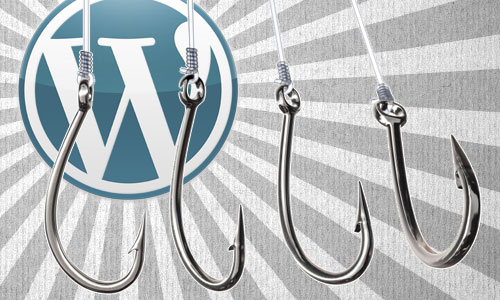
How to use hooks
1.Identify the Hook: Determine whether you need to use an action hook or a filter hook. Action hooks allow you to execute custom code at specific points in WordPress actions, while filter hooks enable you to modify data or content before it is displayed.
2. Create Your Function: Write the custom PHP function that you want to execute when the hook is triggered. This function should contain the code you want to add or modify.
3. Hook Your Function: Use the add_action() function for action hooks or the add_filter() function for filter hooks to attach your custom function to the specific hook you identified. These functions take at least two parameters: the name of the hook and the name of your custom function.
4. Place Your Code: Depending on whether you're working in a theme or a plugin, place your hook and function in the appropriate file. For themes, this could be functions.php, while for plugins, it would be the main plugin file or a separate file within the plugin.
Termomonlogy and Concept
- Hooks: Points in the WordPress code where developers can insert custom code to modify or extend functionality.
- Action Hooks: Points in WordPress actions where custom code can be executed. Actions are events triggered at specific moments during the WordPress execution process.
- Filter Hooks: Points in WordPress where data or content can be modified before it is displayed or used. Filters allow developers to manipulate data by passing it through custom functions.
- Hook Name: The unique identifier for a hook, used when attaching custom functions.
- Callback Function: The custom PHP function that you want to execute when the hook is triggered.
- add_action(): WordPress function used to attach a custom function to an action hook.
- add_filter(): WordPress function used to attach a custom function to a filter hook.
- Priority: An optional parameter when adding hooks that determines the order in which multiple functions attached to the same hook are executed.
- Arguments: Additional parameters passed to the callback function when it’s triggered.
- Remove Action: WordPress function used to remove a previously added action hook.
- Remove Filter: WordPress function used to remove a previously added filter hook.
- Conditional Tags: WordPress functions used within callbacks to determine when to execute the custom code based on specific conditions, such as page type, user role, or other criteria.
- Theme Functions File: The
functions.php
file within a WordPress theme where custom PHP functions and hooks are often added. - Plugin File: The main PHP file of a WordPress plugin where custom functions and hooks are defined.
- Child Theme: A WordPress theme that inherits the functionality and styling of another theme (parent theme), allowing developers to make modifications without directly editing the parent theme files.
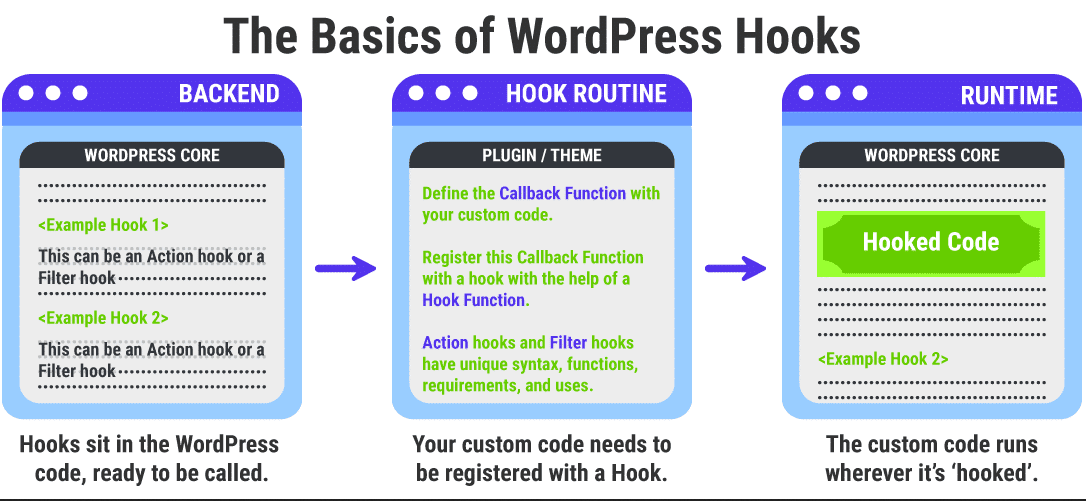
Why hooks exist
* Add/modify functionality to WordPress core
2 Types of hooks
- Actions
- Filter
Action Hooks
Is a php function that is executed at specific points throughout the WordPress core
e.i Creating a widget when WordPress is initializing
Actions allows to run our code at a specific time when WordPress is doing something
Actions
1. do_action() – executes our hooked function
2. add_action() – hooks our custom function (register a callback)
3. remove_action() – un-hooks our custom functions
- do_action():
- Purpose: The
do_action()
function is used to trigger (or fire) an action hook at a specific point in the WordPress codebase. - Syntax:
do_action( $tag, $arg1, $arg2, ... )
$tag
(string): The name of the action hook to be triggered.$arg1, $arg2, ...
(optional): Additional arguments that can be passed to the functions hooked to the action.
- Usage: You use
do_action()
when you want to notify other parts of your code that a particular event has occurred or to execute custom functionality hooked to that action hook. - Example:
php
- Purpose: The
-
-
// Triggering an action hook named 'my_custom_hook'
do_action( 'my_custom_hook', $arg1, $arg2 );
-
- add_action():
- Purpose: The
add_action()
function is used to attach (or register) a custom PHP function to a specific action hook. - Syntax:
add_action( $tag, $function_to_add, $priority, $accepted_args )
$tag
(string): The name of the action hook to which the function is attached.$function_to_add
(callable): The name of the function to be executed when the action hook is triggered.$priority
(int, optional): The priority at which the function should be executed. Lower numbers indicate earlier execution (default is 10).$accepted_args
(int, optional): The number of arguments the function accepts (default is 1).
- Usage: You use
add_action()
when you want to register a custom function to be executed when a specific action hook is triggered. The function will execute whendo_action()
for that hook is called. - Example:
php
- Purpose: The
-
-
// Attaching a custom function 'my_custom_function' to the action hook 'my_custom_hook'
add_action( 'my_custom_hook', 'my_custom_function', 10, 2 );
-
- remove_action():
- Purpose: The
remove_action()
function is used to remove a previously added action hook. - Syntax:
remove_action( $tag, $function_to_remove, $priority )
$tag
(string): The name of the action hook from which the function should be removed.$function_to_remove
(callable): The name of the function that should be removed from the action hook.$priority
(int, optional): The priority at which the function was originally added (if not specified, all occurrences of the function will be removed).
- Usage: You use
remove_action()
when you want to unregister a previously added function from a specific action hook. This is helpful when you need to prevent certain functionality from executing at a particular hook. - Example:
php
- Purpose: The
-
-
// Removing the custom function 'my_custom_function' from the action hook 'my_custom_hook'
remove_action( 'my_custom_hook', 'my_custom_function', 10 );
-
Filter hooks
Filters – Allow us to get and modify WordPress data before It is sent to the database/browser, using our custom functions e.i modify your site title
Filter – Give us access to the data which we can modify and return that data back using our custom functions
Filters (the return a value, always!!!)
1. apply_filter() – executes our hooked function
2. add_filter() – hooks our custom function (register a callback)
3. remove_filter() – removes previously added filter (PS must be the same priority)
- apply_filters():
- Purpose: The
apply_filters()
function is used to apply (or execute) a filter hook to a given data or content. - Syntax:
apply_filters( $tag, $value, $var1, $var2, ... )
$tag
(string): The name of the filter hook to apply.$value
(mixed): The data or content to be filtered.$var1, $var2, ...
(optional): Additional arguments that can be passed to the functions hooked to the filter.
- Usage: You use
apply_filters()
when you want to modify data or content before it’s returned or displayed. The function applies the filter hook, passing the data through any hooked functions, and returns the modified value. - Example:
php
- Purpose: The
-
-
// Applying a filter hook named 'my_custom_filter' to a string value
$filtered_value = apply_filters( 'my_custom_filter', $value, $var1, $var2 );
-
- add_filter():
- Purpose: The
add_filter()
function is used to attach (or register) a custom PHP function to a specific filter hook. - Syntax:
add_filter( $tag, $function_to_add, $priority, $accepted_args )
$tag
(string): The name of the filter hook to which the function is attached.$function_to_add
(callable): The name of the function to be executed when the filter hook is applied.$priority
(int, optional): The priority at which the function should be executed. Lower numbers indicate earlier execution (default is 10).$accepted_args
(int, optional): The number of arguments the function accepts (default is 1).
- Usage: You use
add_filter()
when you want to register a custom function to modify data or content when a specific filter hook is applied. - Example:
php
- Purpose: The
-
-
// Attaching a custom function 'my_custom_filter_function' to the filter hook 'my_custom_filter'
add_filter( 'my_custom_filter', 'my_custom_filter_function', 10, 2 );
-
- remove_filter():
- Purpose: The
remove_filter()
function is used to remove a previously added filter hook. - Syntax:
remove_filter( $tag, $function_to_remove, $priority )
$tag
(string): The name of the filter hook from which the function should be removed.$function_to_remove
(callable): The name of the function that should be removed from the filter hook.$priority
(int, optional): The priority at which the function was originally added (if not specified, all occurrences of the function will be removed).
- Usage: You use
remove_filter()
when you want to unregister a previously added function from a specific filter hook. - Example:
php
- Purpose: The
-
-
// Removing the custom function 'my_custom_filter_function' from the filter hook 'my_custom_filter'
remove_filter( 'my_custom_filter', 'my_custom_filter_function', 10 );
-
Difference between action and filter hooks
WordPress Hooks |
|
Actions | Filters |
Actions are used to run custom functions at a specific point during the execution of WordPress Core. | Filters are used to modify or customize data used by other functions. |
Actions are defined/created by the function do_action( ‘action_name’ ) in the WordPress code. |
Filters are defined/created by the function apply_filters( ‘filter_name’, ‘value_to_be_filtered’ ) in the WordPress code. |
Actions are also called Action hooks. | Filters are also called Filter hooks. |
Actions can only be hooked in with Action functions. E.g. add_action() , remove_action() . |
Filters can only be hooked in with Filter functions. E.g. add_filter() , remove_filter() . |
Action functions need not pass any arguments to their callback functions. | Filter functions need to pass at least one argument to their callback functions. |
Action functions can perform any kind of task, including changing the behavior of how WordPress works. | Filter functions only exist to modify the data passed to them by the filters. |
Action functions should return nothing. However, they can echo the output or interact with the database. |
Filter functions must return their changes as output. Even if a filter function changes nothing, it must still return the unmodified input. |
Actions can execute almost anything, as long as the code is valid. | Filters should work in an isolated manner, so they don’t have any unintended side effects. |
Summary: An action interrupts the regular code execution process to do something with the info it receives, but returns nothing back, and then exits. | Summary: A filter modifies the info it receives, returns it back to the calling hook function, and other functions can use the value it returns. |
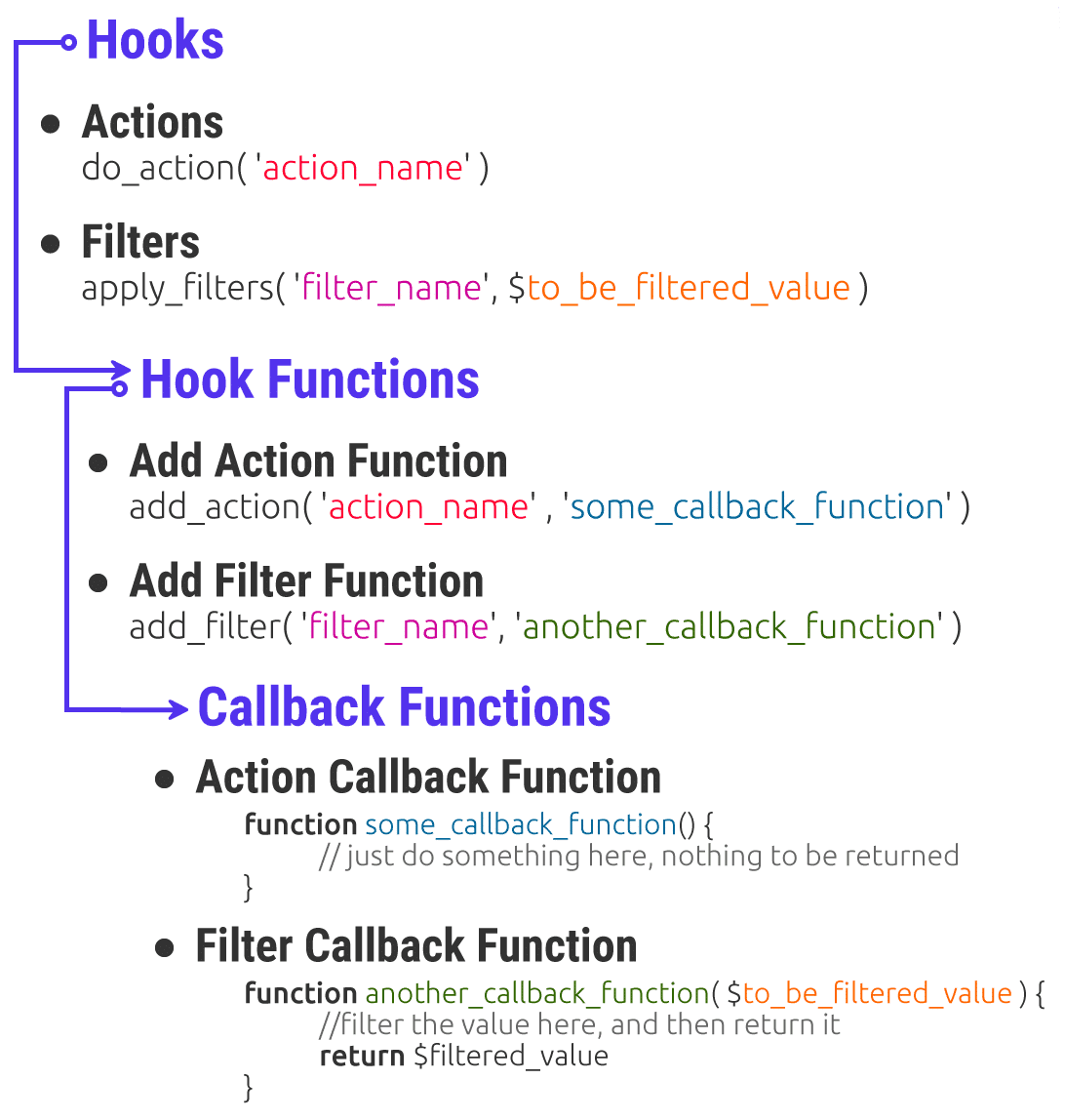
Dissect the functions
Priortity
// priority is set to 9, which is lower than 10, hence it ranks higher
add_action( ‘init’, ‘i_am_high_priority’, 9 );
// if no priority is set, the default value of 10 will be used
add_action( ‘init’, ‘i_am_default_priority’);
// priority is set to 11, which is higher than 11, hence it ranks lower
add_action( ‘init’, ‘i_am_low_priority’, 11 );
Other Action Functions
has_action() : Check if an action has been hooked
do_action() : Define all its default action enabling other functions to hook into them.
do_action_ref_array() : Same as do_action but accepts an array as an argument
did_action() : How many times has an action occurred
remove_all_actions() : Removes everything hooked to an action
doing_action() : Checks whether the specified action is being run to not
Other Filter Functions
has_filter(‘filter_name’, ‘function_to_check’) : Check if a filter hooked by any function
apply_filters() : Define all its default filter enabling other functions to hook into them.
current_filter() : Retrieves the name of the current filter or action being run
remove_filter() : Removes the callback function attached to the specified filter
remove_all_filters() : Removes all the callback functions registered o a filter
doing_filter() : This filter function checks whether the filter specified is being executed at the moment.
Some commonly used hooks
init (action)
wp_enqueue_scripts (action)
add_meta_boxes (action)
save_post (action)
publish_page (action)